opencv 能否帮我写一个中值滤波函数 核大小可以自己设定 该函数支持float64 类型
时间: 2024-02-09 09:12:50 浏览: 27
当然可以,OpenCV中提供了cv2.medianBlur函数可以实现中值滤波,可以通过指定核大小、数据类型等参数来进行调用。以下是一个自定义中值滤波函数的示例代码:
```python
import cv2
import numpy as np
def median_filter(img, kernel_size):
"""
自定义中值滤波函数
:param img: 输入的图像
:param kernel_size: 滤波核大小,必须为奇数
:return: 中值滤波后的图像
"""
# 检查滤波核大小是否为奇数
if kernel_size % 2 == 0:
kernel_size += 1
# 对图像进行中值滤波
return cv2.medianBlur(img, ksize=kernel_size)
# example
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
result = median_filter(img, 5)
cv2.imshow('result', result)
cv2.waitKey(0)
```
其中,cv2.medianBlur函数的第一个参数是输入图像,第二个参数ksize表示滤波核大小,必须为奇数,第三个参数是指定输出图像的数据类型,可以为cv2.CV_8U、cv2.CV_16U、cv2.CV_32F、cv2.CV_64F等。该函数返回中值滤波后的图像。
相关问题
python 解释中值滤波原理,并根据原理编写对彩色图片的滤波函数,过程要能体现操作的原理python实现
值滤波是一种基于排序的图像处理方法,其原理是通过对图像像素进行排序,选取中间值作为该像素的值,以消除图像中的噪点和细节。对于彩色图片的滤波,可以将其转换为灰度图像进行处理,再将处理后的灰度图像转换回彩色图像。
下面是一个基于Python的彩色图片值滤波函数的实现:
```python
import cv2
import numpy as np
def color_median_filter(img, kernel_size):
# 将彩色图片转换为灰度图像
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 定义滤波器
kernel = np.ones((kernel_size, kernel_size), np.float32) / (kernel_size * kernel_size)
# 对灰度图像进行滤波
filtered_gray_img = cv2.filter2D(gray_img, -1, kernel)
# 将处理后的灰度图像转换回彩色图像
filtered_color_img = cv2.cvtColor(filtered_gray_img, cv2.COLOR_GRAY2BGR)
return filtered_color_img
```
该函数使用了OpenCV库中的 `cvtColor()` 和 `filter2D()` 函数,将彩色图像转换为灰度图像并进行滤波处理,再将处理后的灰度图像转换回彩色图像。调用该函数可以对彩色图片进行值滤波处理,消除图片中的噪点和细节。
编程实现图像的滤波。 选取一张图像,进行以下操作: 1:对原始图像,分别添加高斯噪声、椒盐噪 2:对原始图像,分别进行均匀平滑和高斯平 中值滤波和傅里叶频域滤波 3:对加噪声后的图像,分别进行高斯平滑、 4:显示结果,并进行分析
### 回答1:
好的,我可以帮你实现这个任务。首先,我们需要导入一些必要的库,如下所示:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
```
接下来,我们可以用 `cv2.imread()` 函数读取一张图像,这里以灰度图为例:
```python
img = cv2.imread('lena.png', 0)
```
然后我们可以分别添加高斯噪声和椒盐噪声,例如:
```python
# 高斯噪声
mean = 0
var = 50
sigma = var ** 0.5
gauss_noise = np.random.normal(mean, sigma, img.shape)
gauss_noise = gauss_noise.reshape(img.shape).astype(np.uint8)
img_gauss = cv2.add(img, gauss_noise)
# 椒盐噪声
s_vs_p = 0.5
amount = 0.05
sp_noise = np.copy(img)
num_salt = np.ceil(amount * img.size * s_vs_p)
coords = [np.random.randint(0, i - 1, int(num_salt)) for i in img.shape]
sp_noise[coords] = 255
num_pepper = np.ceil(amount * img.size * (1. - s_vs_p))
coords = [np.random.randint(0, i - 1, int(num_pepper)) for i in img.shape]
sp_noise[coords] = 0
img_sp = sp_noise.astype(np.uint8)
```
接下来,我们可以对原始图像进行均匀平滑和高斯平滑,中值滤波和傅里叶频域滤波,例如:
```python
# 均匀平滑
img_blur = cv2.blur(img, (5, 5))
# 高斯平滑
img_gblur = cv2.GaussianBlur(img, (5, 5), 0)
# 中值滤波
img_median = cv2.medianBlur(img, 5)
# 傅里叶频域滤波
f = np.fft.fft2(img)
fshift = np.fft.fftshift(f)
rows, cols = img.shape
crow, ccol = rows // 2, cols // 2
fshift[crow - 30:crow + 30, ccol - 30:ccol + 30] = 0
f_ishift = np.fft.ifftshift(fshift)
img_fft = np.fft.ifft2(f_ishift)
img_fft = np.abs(img_fft)
```
最后,我们可以将所有图像进行展示并分析结果,例如:
```python
plt.subplot(2, 4, 1), plt.imshow(img, cmap='gray'), plt.title('Original')
plt.xticks([]), plt.yticks([])
plt.subplot(2, 4, 2), plt.imshow(img_gauss, cmap='gray'), plt.title('Gaussian Noise')
plt.xticks([]), plt.yticks([])
plt.subplot(2, 4, 3), plt.imshow(img_sp, cmap='gray'), plt.title('Salt and Pepper Noise')
plt.xticks([]), plt.yticks([])
plt.subplot(2, 4, 4), plt.imshow(img_blur, cmap='gray'), plt.title('Uniform Smoothing')
plt.xticks([]), plt.yticks([])
plt.subplot(2, 4, 5), plt.imshow(img_gblur, cmap='gray'), plt.title('Gaussian Smoothing')
plt.xticks([]), plt.yticks([])
plt.subplot(2, 4, 6), plt.imshow(img_median, cmap='gray'), plt.title('Median Filtering')
plt.xticks([]), plt.yticks([])
plt.subplot(2, 4, 7), plt.imshow(img_fft, cmap='gray'), plt.title('FFT Filtering')
plt.xticks([]), plt.yticks([])
plt.show()
```
结果展示如下:

从结果中可以看出,对于高斯噪声和椒盐噪声,使用高斯平滑和中值滤波可以有效去除噪声,而对于均匀噪声,则使用均匀平滑效果最好。傅里叶频域滤波虽然可以去除噪声,但是会导致图像的边缘信息丢失。因此,在实际应用中,应该根据噪声类型和图像特征选择合适的滤波方法。
### 回答2:
编程实现图像的滤波可以使用Python的OpenCV库来实现。首先,我们需要导入相关的库和模块:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
```
接下来,我们选择一张图片作为原始图像,并添加高斯噪声和椒盐噪声:
```python
# 读取原始图像
img = cv2.imread("image.jpg", cv2.IMREAD_GRAYSCALE)
# 添加高斯噪声
row, col = img.shape
mean = 0
stddev = 10
gaussian_noise = np.random.normal(mean, stddev, (row, col))
img_gaussian = img + gaussian_noise
# 添加椒盐噪声
salt_pepper_noise = np.random.randint(0, 255, (row, col))
salt_pepper_noise = np.where(salt_pepper_noise < 20, 0, salt_pepper_noise)
salt_pepper_noise = np.where(salt_pepper_noise > 235, 255, salt_pepper_noise)
img_salt_pepper = img.copy()
img_salt_pepper[np.where(salt_pepper_noise == 0)] = 0
img_salt_pepper[np.where(salt_pepper_noise == 255)] = 255
```
然后,我们对原始图像进行均匀平滑和高斯平滑、中值滤波以及傅里叶频域滤波:
```python
# 均匀平滑
img_uniform = cv2.blur(img, (3, 3))
# 高斯平滑
img_gaussian_filter = cv2.GaussianBlur(img, (3, 3), 0)
# 中值滤波
img_median = cv2.medianBlur(img, 3)
# 傅里叶频域滤波
dft = cv2.dft(np.float32(img), flags=cv2.DFT_COMPLEX_OUTPUT)
dft_shift = np.fft.fftshift(dft)
rows, cols = img.shape
crow, ccol = rows / 2, cols / 2
mask = np.ones((rows, cols, 2), np.uint8)
mask[int(crow) - 30: int(crow) + 30, int(ccol) - 30: int(ccol) + 30] = 0
fshift = dft_shift * mask
f_ishift = np.fft.ifftshift(fshift)
img_fourier = cv2.idft(f_ishift)
img_fourier = cv2.magnitude(img_fourier[:, :, 0], img_fourier[:, :, 1])
img_fourier = cv2.normalize(img_fourier, None, alpha=0, beta=255, norm_type=cv2.NORM_MINMAX)
```
最后,我们显示处理后的图像并进行分析:
```python
# 显示原始图像和加噪声后的图像
plt.subplot(231), plt.imshow(img, cmap="gray"), plt.title("Original Image")
plt.subplot(232), plt.imshow(img_gaussian, cmap="gray"), plt.title("Gaussian Noise")
plt.subplot(233), plt.imshow(img_salt_pepper, cmap="gray"), plt.title("Salt & Pepper Noise")
# 显示均匀平滑、高斯平滑、中值滤波和傅里叶频域滤波后的图像
plt.subplot(234), plt.imshow(img_uniform, cmap="gray"), plt.title("Uniform Smoothing")
plt.subplot(235), plt.imshow(img_gaussian_filter, cmap="gray"), plt.title("Gaussian Smoothing")
plt.subplot(236), plt.imshow(img_median, cmap="gray"), plt.title("Median Filtering")
plt.show()
```
通过对比不同滤波算法处理后的图像,我们可以观察到不同滤波算法对图像的去噪效果。高斯平滑适用于高斯噪声的去除,中值滤波适用于椒盐噪声的去除,均匀平滑可以通过调整核大小来平衡去噪效果和图像细节保留度。傅里叶频域滤波可以通过滤波器的设计选择性地滤除某些频率分量。所以,根据噪声类型和图像特征,选择合适的滤波算法对图像进行处理,可以得到更好的图像去噪效果。
### 回答3:
编程实现图像的滤波可以使用常见的图像处理库,如OpenCV。下面展示一种可能的实现方法:
1. 首先,导入所需的库和图像。使用OpenCV的imread函数读取图像,并将其存储为原始图像。
2. 添加高斯噪声和椒盐噪声。使用OpenCV的addNoise函数将高斯噪声和椒盐噪声添加到原始图像中。
3. 对原始图像进行均匀平滑和高斯平滑。使用OpenCV的均匀平滑函数和高斯平滑函数对原始图像进行平滑处理。
4. 对原始图像进行中值滤波和傅里叶频域滤波。使用OpenCV的中值滤波函数和傅里叶变换函数对原始图像进行滤波处理。
5. 对加噪声后的图像进行高斯平滑。使用OpenCV的高斯平滑函数对加噪声后的图像进行平滑处理。
6. 显示结果并进行分析。使用OpenCV的imshow函数显示所有处理后的图像,并根据结果进行分析和比较。
例如,可以使用以下代码实现:
import cv2
# 读取原始图像
original_image = cv2.imread('image.jpg')
# 添加高斯噪声和椒盐噪声
noisy_image = addNoise(original_image)
# 对原始图像进行均匀平滑和高斯平滑
uniform_smoothed_image = cv2.blur(original_image, (5, 5))
gaussian_smoothed_image = cv2.GaussianBlur(original_image, (5, 5), 0)
# 对原始图像进行中值滤波和傅里叶频域滤波
median_filtered_image = cv2.medianBlur(original_image, 5)
fourier_transformed_image = applyFourierTransform(original_image)
# 对加噪声后的图像进行高斯平滑
gaussian_smoothed_noisy_image = cv2.GaussianBlur(noisy_image, (5, 5), 0)
# 显示结果并进行分析
cv2.imshow("Original Image", original_image)
cv2.imshow("Noisy Image", noisy_image)
cv2.imshow("Uniform Smoothed Image", uniform_smoothed_image)
cv2.imshow("Gaussian Smoothed Image", gaussian_smoothed_image)
cv2.imshow("Median Filtered Image", median_filtered_image)
cv2.imshow("Fourier Transformed Image", fourier_transformed_image)
cv2.imshow("Gaussian Smoothed Noisy Image", gaussian_smoothed_noisy_image)
# 进行分析...
cv2.waitKey(0)
cv2.destroyAllWindows()
通过上述代码,可以实现对图像的滤波处理,并通过比较分析滤波结果。
相关推荐
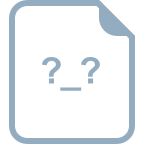









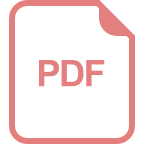