C++获取TEXTBOX的字符串
时间: 2023-10-23 11:12:26 浏览: 49
在Windows平台下,可以使用WinAPI中的GetWindowText函数来获取文本框的字符串。该函数的原型如下:
```c++
int GetWindowText(
HWND hWnd,
LPTSTR lpString,
int nMaxCount
);
```
其中,hWnd参数是文本框的句柄;lpString参数是接收文本框字符串的指针;nMaxCount参数是接收字符串的缓冲区长度。
以下是一个示例代码:
```c++
#include <Windows.h>
#include <iostream>
int main()
{
HWND hwnd = GetForegroundWindow(); // 获取当前窗口的句柄
TCHAR buffer[1024] = { 0 };
int length = GetWindowText(hwnd, buffer, sizeof(buffer) / sizeof(TCHAR)); // 获取文本框字符串
std::wcout << buffer << std::endl; // 输出文本框字符串
return 0;
}
```
注意,GetWindowText函数只能获取普通的文本框字符串,如果文本框中包含其它类型的数据(如图片、超链接等),则无法获取。如果需要获取更复杂的文本框内容,可以考虑使用其它第三方库或框架。
相关问题
winform 获取Textbox字符串
在WinForm中,可以通过TextBox控件的Text属性来获取文本框中的字符串。
```csharp
string text = textBox.Text;
```
上述代码将文本框中的文本内容赋值给text变量。需要注意的是,如果文本框中没有任何内容,Text属性将返回一个空字符串。
如果需要在获取文本框内容之前对其进行一些处理,例如去除前后空格或者转换大小写等操作,可以使用Trim方法和ToUpper或ToLower方法。例如:
```csharp
string text = textBox.Text.Trim().ToUpper();
```
上述代码将文本框中的文本内容去除前后空格,并将其转换为大写字母后赋值给text变量。需要注意的是,对文本框内容进行处理时,应该根据实际需要选择合适的处理方式。
C#获取textbox字符串的值
在C#中获取textbox字符串的值可以通过访问textbox的Text属性来实现。例如,如果你有一个名为tb_QRCode的textbox控件,你可以使用tb_QRCode.Text来获取该控件中的字符串值。在你的代码中,步骤三中的Tb_QRCode_TextChanged方法中的tb_QRCode.Text就是获取textbox字符串的值的地方。\[1\] 你可以在TextChanged事件中使用这个值进行进一步的处理。
#### 引用[.reference_title]
- *1* *2* [C# textbox控件输入字符串触发回车事件](https://blog.csdn.net/tiegenZ/article/details/104990098)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [关于c#:输入字符串的格式不正确](https://blog.csdn.net/weixin_42514627/article/details/117036758)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
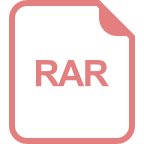
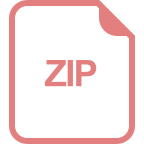













