android studio实现点击一个标签展开内容
时间: 2023-07-18 14:10:39 浏览: 165
如果你想在 Android Studio 中实现点击一个标签展开内容的功能,可以考虑使用 ExpandableListView 组件。这个组件可以让你在一个列表中展示可展开的子项,每个子项可以包含一个或多个子视图。
下面是一个简单的步骤指南:
1. 在你的布局文件中添加 ExpandableListView 组件:
```xml
<ExpandableListView
android:id="@+id/my_expandable_list"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
```
2. 在你的 Activity 或 Fragment 中获取 ExpandableListView 对象,并设置适配器:
```java
ExpandableListView expandableListView = findViewById(R.id.my_expandable_list);
MyExpandableListAdapter adapter = new MyExpandableListAdapter(this, groups, children);
expandableListView.setAdapter(adapter);
```
其中,`MyExpandableListAdapter` 是你自己定义的一个适配器类,`groups` 和 `children` 分别是一个字符串数组,用于存储父项和子项的数据。
3. 在适配器类中实现 `getGroupView` 和 `getChildView` 方法,用于生成父项和子项的视图:
```java
public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = LayoutInflater.from(context).inflate(R.layout.group_item_layout, parent, false);
}
TextView groupTextView = convertView.findViewById(R.id.group_text_view);
groupTextView.setText(groups[groupPosition]);
return convertView;
}
public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = LayoutInflater.from(context).inflate(R.layout.child_item_layout, parent, false);
}
TextView childTextView = convertView.findViewById(R.id.child_text_view);
childTextView.setText(children[groupPosition][childPosition]);
return convertView;
}
```
其中,`group_item_layout` 和 `child_item_layout` 分别是你定义的父项和子项的布局文件。
4. 在适配器类中实现 `isChildSelectable` 和 `onChildClick` 方法,用于处理子项的点击事件:
```java
public boolean isChildSelectable(int groupPosition, int childPosition) {
return true;
}
public boolean onChildClick(ExpandableListView parent, View v, int groupPosition, int childPosition, long id) {
// 处理子项的点击事件
return true;
}
```
当用户点击子项时,`onChildClick` 方法会被调用,你可以在这个方法中处理子项的点击事件,比如展开或关闭子项的内容。
希望这个步骤指南能对你有所帮助!
阅读全文
相关推荐
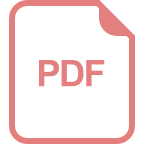
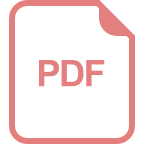

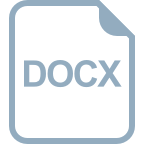
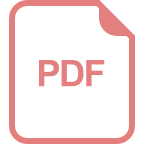
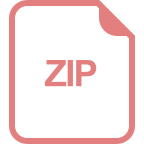
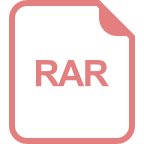
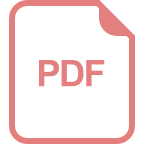
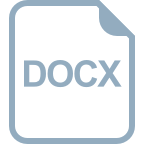
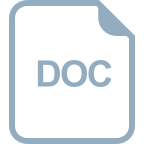






