c++操作mongodb数据库,读取数据,并修改当前数据,增加新的列名。写出详细的代码
时间: 2024-09-21 13:11:27 浏览: 53
在C++中,直接操作MongoDB数据库通常不是最直观的方式,因为C++的标准库并没有内置的支持。但是,你可以使用像`mongo-cxx-driver`这样的第三方库来实现这个需求,它提供了一个C++驱动程序来与MongoDB交互。
首先,你需要安装`mongo-cxx-driver`,可以通过包管理器(如Ubuntu的`apt-get`或Conan等构建工具)或从GitHub下载源码编译。
下面是一个简单的示例,展示了如何使用`mongo-cxx-driver`连接到MongoDB、读取数据、更新文档以及添加新字段:
```cpp
#include <mongocxx/client.hpp>
#include <mongocxx/database.hpp>
#include <mongocxx/collection.hpp>
using namespace mongocxx;
int main() {
// 创建MongoDB客户端
client client{ uri{} };
try {
// 连接到集合
database db = client["your_database"];
collection coll = db["your_collection"];
// 查询文档并获取一个读操作符
bsoncxx::document::view query = bsoncxx::builder::stream::document{}
<< "query_field" << "some_value"
.finalize();
auto cursor = coll.find(query);
// 遍历查询结果
for (auto& doc : cursor) {
// 更新文档并添加新字段
bsoncxx::builder::stream::document update = bsoncxx::builder::stream::document{}
<< "$set" << bsoncxx::builder::stream::open_document
<< "new_column_name" << doc.view()["existing_column_name"]
<< bsoncxx::builder::stream::close_document;
coll.update_one(doc.view(), update.view());
// 如果你想打印出修改后的文档,可以这样做
std::cout << "Updated document: \n" << bsoncxx::to_json(update.view()) << std::endl;
}
} catch (std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
记得替换`"your_database"`和`"your_collection"`为你实际的数据库和集合名称,以及`query_field`和`existing_column_name`为你要查询和更新的实际字段。
相关推荐
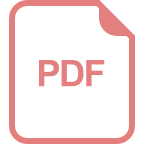
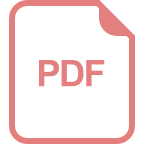
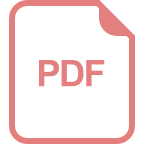














