在C# winform中实现对VerticalLineAnnotation拖动事件的监听
时间: 2024-09-12 22:09:54 浏览: 48
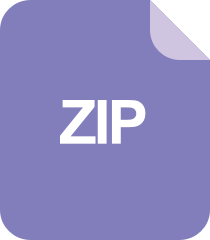
C#实现Winform实现区域截屏
在C#的WinForms应用程序中,如果你使用的是.NET Framework 4.0或更高版本,可能会用到System.Windows.Forms.DataVisualization命名空间下的Chart控件。在Chart控件中,Annotations用于在图表上添加注解,比如标记线。VerticalLineAnnotation是一种垂直线注解。
要实现对VerticalLineAnnotation拖动事件的监听,你需要首先为VerticalLineAnnotation添加一个鼠标事件处理器。然而,标准的VerticalLineAnnotation并不直接支持拖动事件。为了实现这一功能,你通常需要自定义一个继承自VerticalLineAnnotation的类,并且重写其鼠标事件处理逻辑。
以下是一个简单的示例,展示如何创建一个自定义的VerticalLineAnnotation类,并实现拖动事件的监听:
```csharp
using System;
using System.Windows.Forms.DataVisualization.Charting;
public class DraggableVerticalLineAnnotation : VerticalLineAnnotation
{
private bool isDragging = false;
private float lastX = 0;
public DraggableVerticalLineAnnotation()
{
// 这里可以设置默认属性
}
protected override void OnMouseDown(MouseEventArgs e)
{
base.OnMouseDown(e);
// 检查是否点击了垂直线
if (e.Button == MouseButtons.Left && IsPositionOnAnnotation(e.X, e.Y))
{
isDragging = true;
lastX = e.X;
}
}
protected override void OnMouseMove(MouseEventArgs e)
{
base.OnMouseMove(e);
if (isDragging)
{
// 计算新的X位置
float newX = e.X;
float deltaX = newX - lastX;
lastX = newX;
// 更新注解位置
Annotation.X = Annotation.X + deltaX;
this.Chart.Refresh();
// 可以调用一个函数来调整图表其他元素的位置,以保持一致性
AdjustOtherElements();
}
}
protected override void OnMouseUp(MouseEventArgs e)
{
base.OnMouseUp(e);
if (e.Button == MouseButtons.Left)
{
isDragging = false;
}
}
private bool IsPositionOnAnnotation(int x, int y)
{
// 判断点击位置是否在注解的垂直线范围内
// 实现细节略
}
private void AdjustOtherElements()
{
// 根据需要调整其他图表元素的位置
// 实现细节略
}
}
```
在上面的代码中,我们重写了`OnMouseDown`、`OnMouseMove`和`OnMouseUp`方法来处理鼠标事件。当用户按下鼠标左键并且鼠标位置在垂直线上时,开始拖动操作。在`OnMouseMove`中计算新的位置,并更新注解的位置。当用户释放鼠标时,结束拖动操作。
要使用这个自定义的VerticalLineAnnotation,你可以在你的WinForms界面设计器中添加一个Chart控件,并通过代码添加这个自定义的注解。
请注意,上述代码只是一个示例,你需要根据实际情况进行调整和完善。特别是`IsPositionOnAnnotation`和`AdjustOtherElements`方法需要根据你的图表的具体逻辑来实现。
阅读全文
相关推荐
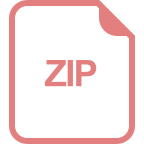
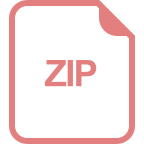
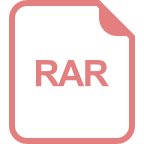
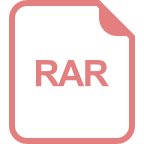
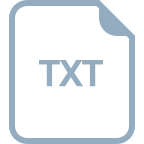
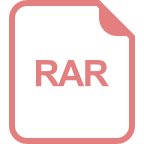
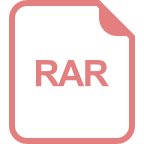
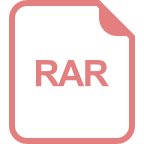
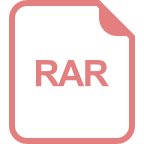
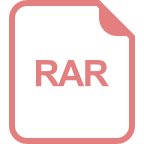
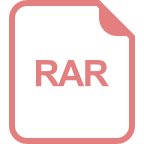
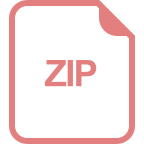
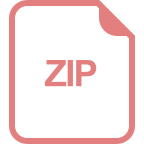
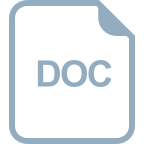
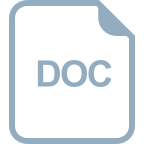
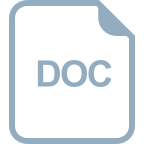
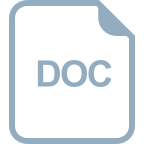

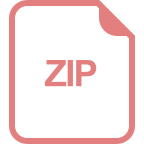