用python写一个程序实动态现模拟 一个长方形的中心在贝塞尔曲线上沿着这条曲线的切线方向运动
时间: 2023-12-03 10:05:32 浏览: 76
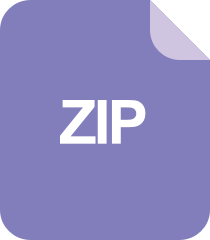
Python实现简易的交互式绘制贝塞尔曲线程序,可拼接,可拖动
可以使用 Python 中的 matplotlib 库来实现这个动态模拟。下面是一个示例程序,可以模拟一个长方形的中心沿着贝塞尔曲线切线方向运动:
```python
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
# 贝塞尔曲线控制点
control_points = np.array([[0, 0], [1, 3], [2, -3], [3, 0]])
# 计算贝塞尔曲线上的点
def compute_bezier(t):
n = len(control_points) - 1
result = np.zeros(2)
for i in range(n + 1):
result += control_points[i] * binomial_coefficient(n, i) * (1 - t)**(n - i) * t**i
return result
# 计算二项式系数
def binomial_coefficient(n, k):
return np.math.factorial(n) / (np.math.factorial(k) * np.math.factorial(n - k))
# 初始化图形
fig, ax = plt.subplots()
ax.set_xlim(-1, 4)
ax.set_ylim(-4, 4)
# 绘制贝塞尔曲线
t_values = np.linspace(0, 1, 100)
curve_points = np.array([compute_bezier(t) for t in t_values])
curve_line, = ax.plot(curve_points[:, 0], curve_points[:, 1], 'b-')
# 绘制长方形
rectangle = plt.Rectangle((0, 0), 0.2, 0.5, fc='r')
ax.add_patch(rectangle)
# 动画更新函数
def update(frame):
t = frame / 100
# 计算当前点在曲线上的位置和切线方向
p = compute_bezier(t)
tangent = compute_bezier(t + 0.01) - p
angle = np.arctan2(tangent[1], tangent[0])
# 更新长方形的位置和旋转角度
rectangle.set_xy(p - np.array([0.1, 0.25]))
rectangle.set_angle(np.degrees(angle))
return [rectangle]
# 创建动画
ani = animation.FuncAnimation(fig, update, frames=100, interval=50, blit=True)
# 显示动画
plt.show()
```
这段代码使用了 numpy 和 matplotlib 库。首先,定义了贝塞尔曲线的控制点 `control_points`,然后实现了计算贝塞尔曲线上的点的函数 `compute_bezier`,以及计算二项式系数的函数 `binomial_coefficient`。
接下来,创建了一个图形窗口,并绘制了贝塞尔曲线和一个红色长方形。然后,定义了动画更新函数 `update`,在每一帧中根据当前时间参数 t 计算曲线上的位置和切线方向,更新长方形的位置和旋转角度。
最后,使用 `animation.FuncAnimation` 创建了动画,并通过 `plt.show()` 显示出来。可以根据需要调整动画的帧数、帧间隔等参数来获得更好的效果。
阅读全文
相关推荐
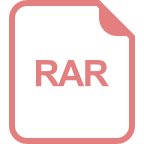










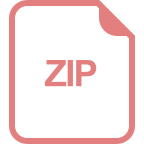