无线电 iq 数据通过 udp 传输,qt 实现,接收端收到数据后存入文件中,请举例
时间: 2024-11-06 08:24:43 浏览: 30
在Qt中,使用UDP(User Datagram Protocol)协议处理无线电IQ(In-phase and Quadrature)数据并将其存储到文件中,首先你需要设置一个UDP socket监听器来接收数据,然后解析接收到的数据并将它们写入文件。以下是一个简单的示例代码:
```cpp
#include <QObject>
#include <QThread>
#include <QUdpSocket>
#include <QDataStream>
#include <QFile>
#include <QDir>
class UDPReceiver : public QObject
{
Q_OBJECT
public slots:
void startReceiving();
void stopReceiving();
private:
QUdpSocket *udpSocket;
QDir dir;
QFile file;
signals:
void dataReceived(const QByteArray &data);
private:
void receiveLoop();
};
void UDPReceiver::startReceiving()
{
if (!udpSocket->isValid()) {
qDebug() << "Starting UDP receiver";
udpSocket = new QUdpSocket(this);
connect(udpSocket, SIGNAL(error(QAbstractSocket::SocketError)), this, SLOT(handleError()));
connect(udpSocket, SIGNAL(readyRead()), this, SLOT(receiveData()));
if (!udpSocket->bind("0.0.0.0", 5000)) { // Listen on any available port
qCritical() << "Failed to bind UDP socket";
return;
}
thread = new QThread(this);
moveToThread(thread);
thread->start();
}
}
void UDPReceiver::stopReceiving()
{
if (thread && thread->isRunning()) {
disconnect(udpSocket, NULL, this, NULL);
emit dataReceived(nullptr); // Signal to stop receiving in the thread
thread->quit();
thread->wait(); // Wait for the thread to finish
delete thread;
thread = nullptr;
delete udpSocket;
}
}
void UDPReceiver::receiveData()
{
QByteArray buffer;
while (udpSocket->hasPendingDatagrams()) {
buffer += udpSocket->readDatagram(1024);
emit dataReceived(buffer); // Emit signal with received data
}
}
void UDPReceiver::handleError()
{
qWarning() << "UDP error: " << static_cast<QAbstractSocket::SocketError>(udpSocket->error());
}
// In your main application or a separate class where you use UDPReceiver
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
UDPReceiver receiver;
receiver.startReceiving();
// ... Your main loop here ...
receiver.stopReceiving(); // Stop receiving when needed
return app.exec();
}
```
在这个例子中,`UDPReceiver`类负责创建一个UDP套接字,开始监听,并在接收到数据时触发信号。当停止接收时,你可以关闭套接字,并在主线程中调用`receiver.stopReceiving()`。
每次有新的数据到达,`dataReceived`信号会被发射,你可以在这里处理数据,例如:
```cpp
// 在主窗口或者其他接收者类里
connect(receiver, &UDPReceiver::dataReceived, this, [receiver](const QByteArray &data) {
if (data.isEmpty()) {
receiver.stopReceiving(); // If there's no more data, stop receiving
return;
}
// Parse IQ data
QDataStream stream(data);
// ... Process IQ data here ...
// Save to a file, assuming 'iqFile' is already open in write mode
qint64 bytesWritten = stream.writeTo(iqFile);
if (bytesWritten != data.size()) {
qCritical() << "Failed to write IQ data to file";
}
});
```
这个例子展示了基本的接收、解析和保存过程,实际应用中可能还需要根据具体的数据格式来解析IQ数据。同时,为了更好地处理网络错误和异常情况,你可能需要添加更多的错误检查和恢复机制。
阅读全文
相关推荐
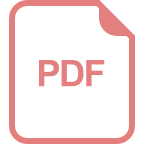
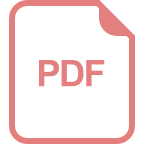
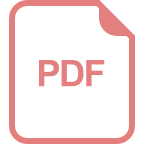
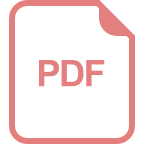
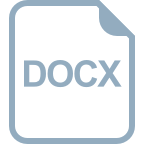
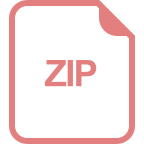
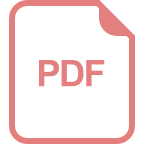
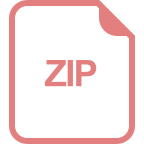
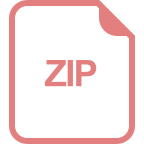
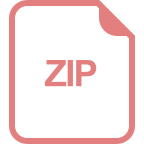
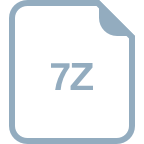
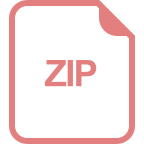
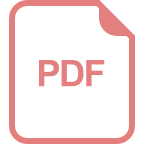
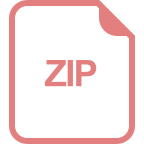
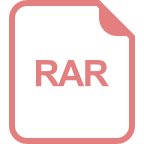
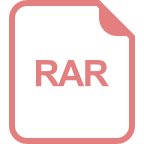