print(img_file) for img_file in img_files: img_path = os.path.join(image_folder_path,img_file) img = cv2.imread(img_path) barcodes = pyzbar.decode(img) for barcode in barcodes: if barcode.type == 'QRCODE': qr_data = barcode.data.decode("utf-8") qr_codes_found.append((img_file, qr_data)) with open(output_file_name,'w') as f: for file_name,qr_content in qr_codes_found: file_name = os.path.splitext(file_name)[0] # 去掉文件名的扩展名 f.write(f"{file_name}: {qr_content}\n") if __name__ == "__main__": image_folder_path = '/root/pictures' output_file_name = 'qr_codes_found.txt' main(image_folder_path,output_file_name)
时间: 2024-04-19 22:24:32 浏览: 140
这段代码是用于在给定的图像文件夹中查找并解码所有的QR码,并将结果保存在一个文本文件中。代码使用了`pyzbar`库来解码QR码,`cv2`库来读取图像。下面是代码的执行步骤:
1. 打印`img_file`变量的值。
2. 对于图像文件夹中的每个图像文件,获取图像文件的路径。
3. 使用`cv2.imread`函数读取图像。
4. 使用`pyzbar.decode`函数解码图像中的所有条形码。
5. 对于每个解码出的条形码,检查其类型是否为QR码。
6. 如果是QR码,将解码出的数据转换为UTF-8编码,并将图像文件名和QR码数据添加到`qr_codes_found`列表中。
7. 使用`open`函数创建一个名为`output_file_name`的文本文件,并以写入模式打开。
8. 对于`qr_codes_found`列表中的每个图像文件名和QR码数据,去掉文件名的扩展名,并将结果以"文件名: QR码内容"的格式写入文本文件中。
9. 如果以上代码是直接执行的(而不是被导入为模块),则将图像文件夹路径和输出文件名作为参数传递给`main`函数。
请注意,这只是一个示例代码,并不完整。您需要根据实际需求进行适当的修改和补充。
相关问题
#!/usr/bin/env python2.7 # -*- coding: UTF-8 -*- import rospy from sensor_msgs.msg import Image from cv_bridge import CvBridge import cv2 import os from pyzbar import pyzbar def image_callback(msg): # 将ROS图像消息转换为OpenCV图像 bridge = CvBridge() frame = bridge.imgmsg_to_cv2(msg, desired_encoding='bgr8') # 执行生成文本的逻辑 image_folder_path = '/root/Pictures' output_file_name = '/root/Pictures/qr_codes_found.txt' main(image_folder_path, output_file_name) def main(image_folder_path, output_file_name): img_files = [f for f in os.listdir(image_folder_path) if f.endswith('.png')] qr_codes_found = [] print("Image files:") for img_file in img_files: print(img_file) for img_file in img_files: img_path = os.path.join(image_folder_path, img_file) img = cv2.imread(img_path) barcodes = pyzbar.decode(img) for barcode in barcodes: if barcode.type == 'QRCODE': qr_data = barcode.data.decode("utf-8") qr_codes_found.append((img_file, qr_data)) unique_qr_codes = [] for file_name, qr_content in qr_codes_found: if qr_content not in unique_qr_codes: unique_qr_codes.append(qr_content) with open(output_file_name, 'w') as f: for qr_content in unique_qr_codes: f.write("{}\n".format(qr_content)) if __name__ == '__main__': rospy.init_node('text_generation_node') # 创建一个订阅器订阅图像消息 rospy.Subscriber('processed_image', Image, image_callback) rospy.spin() 如何让这个代码生成的文本变为excel文件
要将生成的文本内容保存为 Excel 文件,您可以使用 Python 的 openpyxl 库来实现。下面是修改后的代码,可将生成的文本保存为 Excel 文件:
```python
#!/usr/bin/env python2.7
# -*- coding: UTF-8 -*-
import rospy
from sensor_msgs.msg import Image
from cv_bridge import CvBridge
import cv2
import os
from pyzbar import pyzbar
from openpyxl import Workbook
def image_callback(msg):
# 将ROS图像消息转换为OpenCV图像
bridge = CvBridge()
frame = bridge.imgmsg_to_cv2(msg, desired_encoding='bgr8')
# 执行生成文本的逻辑
image_folder_path = '/root/Pictures'
output_file_name = '/root/Pictures/qr_codes_found.xlsx'
main(image_folder_path, output_file_name)
def main(image_folder_path, output_file_name):
img_files = [f for f in os.listdir(image_folder_path) if f.endswith('.png')]
qr_codes_found = []
print("Image files:")
for img_file in img_files:
print(img_file)
for img_file in img_files:
img_path = os.path.join(image_folder_path, img_file)
img = cv2.imread(img_path)
barcodes = pyzbar.decode(img)
for barcode in barcodes:
if barcode.type == 'QRCODE':
qr_data = barcode.data.decode("utf-8")
qr_codes_found.append((img_file, qr_data))
unique_qr_codes = []
for file_name, qr_content in qr_codes_found:
if qr_content not in unique_qr_codes:
unique_qr_codes.append(qr_content)
# 创建一个新的工作簿
wb = Workbook()
# 获取默认的工作表
sheet = wb.active
# 将数据写入工作表
for i, qr_content in enumerate(unique_qr_codes, start=1):
sheet.cell(row=i, column=1).value = qr_content
# 保存工作簿为Excel文件
wb.save(output_file_name)
if __name__ == '__main__':
rospy.init_node('text_generation_node')
# 创建一个订阅器订阅图像消息
rospy.Subscriber('processed_image', Image, image_callback)
rospy.spin()
```
在上面的代码中,我们使用 openpyxl 库来创建一个新的工作簿,并将数据写入工作表中。最后,我们使用 save() 方法将工作簿保存为 Excel 文件。
请确保已安装 openpyxl 库,可以使用以下命令进行安装:
```
pip install openpyxl
```
请根据您的需求修改文件路径和其他部分。
'''截取图片的右半部分''' import cv2 import os def merge_jpg_to_mp4(folder_path, output_file): # 获取文件夹下所有的 JPG 文件 jpg_files = [file for file in os.listdir(folder_path) if file.lower().endswith('.jpg')] jpg_files.sort() # 按文件名排序 # 读取第一张图片,获取尺寸信息 first_image_path = os.path.join(folder_path, jpg_files[0]) first_image = cv2.imread(first_image_path) height, width, _ = first_image.shape width = width // 2 print(height, width) # 创建 VideoWriter 对象 fourcc = cv2.VideoWriter_fourcc(*'mp4v') video_writer = cv2.VideoWriter(output_file, fourcc, 20, (width, height)) # 逐个读取 JPG 文件并写入视频 for jpg_file in jpg_files: image_path = os.path.join(folder_path, jpg_file) image = cv2.imread(image_path) # print(type(image)) width_begin = (image.shape[1] - image.shape[1]//2) image1 = image[:,width_begin:,:] print(image1.shape) # cv2.imshow('img',image) # cv2.waitKey(0) # break video_writer.write(image1) # 释放资源 video_writer.release() # 指定文件夹路径和输出文件名 folder_path = './video/' output_file = 'output3.mp4' # 合并文件夹下的所有 JPG 文件为一个 MP4 文件 merge_jpg_to_mp4(folder_path, output_file)
这段代码是用 Python 的 OpenCV 库实现的,目的是将一个文件夹下的 JPG 图片合成为一个 MP4 视频文件。具体实现步骤如下:
1. 获取文件夹下所有的 JPG 文件,并按照文件名排序。
2. 读取第一张 JPG 图片,获取其尺寸信息,并将其宽度减半,得到视频的宽度。
3. 创建 VideoWriter 对象,指定输出的文件名、编码格式、帧率和视频尺寸。
4. 逐个读取 JPG 文件,并将其右半部分截取下来。
5. 将截取后的图片写入视频。
6. 释放资源。
需要注意的是,由于代码中使用了 cv2.imshow 和 cv2.waitKey,因此需要在运行该代码时添加相应的 GUI 环境,否则会出现错误。
阅读全文
相关推荐
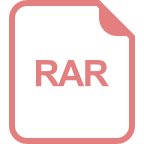
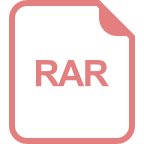
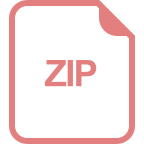





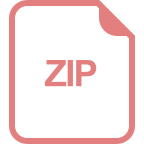
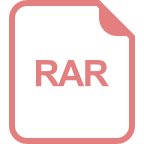