python英文的词云图
时间: 2023-11-01 20:08:33 浏览: 141
To create a word cloud in Python, you can use the "wordcloud" library. Here is a simple example:
```python
from wordcloud import WordCloudimport matplotlib.pyplot as plt
# Your text data
text = "This is an example text for creating a word cloud in Python."
# Generate the word cloud
wordcloud = WordCloud().generate(text)
# Display the word cloud
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.show()
```
This code will generate a word cloud based on the input text using default settings. You can customize the appearance and behavior of the word cloud by specifying different parameters.
相关问题
Python显示词云图
要显示词云图,可以使用Python的第三方库wordcloud。首先需要用pip安装该库,安装命令为:
pip install wordcloud
接下来就可以使用wordcloud创建词云图了。以下是一个简单的例子:
```python
import jieba
from wordcloud import WordCloud
text = "Python is a widely used high-level programming language for general-purpose programming, created by Guido van Rossum and first released in 1991. An interpreted language, Python has a design philosophy which emphasizes code readability (notably using whitespace indentation to delimit code blocks rather than curly brackets or keywords), and a syntax which allows programmers to express concepts in fewer lines of code than might be possible in languages such as C++ or Java. The language provides constructs intended to enable writing clear programs on both a small and large scale."
# 使用jieba分词
wordlist = jieba.cut(text, cut_all=True)
# 用空格连接分词结果,得到一个字符串
word_str = ' '.join(wordlist)
# 生成词云图
wc = WordCloud(background_color='white', max_words=2000, font_path='msyh.ttc')
wc.generate(word_str)
# 显示词云图
import matplotlib.pyplot as plt
plt.imshow(wc)
plt.axis('off')
plt.show()
```
以上代码将生成一个基本的英文词云图。中文词云图的生成也类似,只需替换掉分词器即可。
Python英语词云制作
Python中可以使用一些库如wordcloud和matplotlib等来创建英语词云,这是一种可视化文本数据的方法,其中常用单词按照频率以更大的字体显示在图片上。以下是一个简单的步骤:
1. 安装所需库:首先,你需要安装`wordcloud`和`matplotlib`。你可以使用pip命令来安装它们:
```
pip install wordcloud matplotlib
```
2. 导入库并读取文本:导入`wordcloud`和`matplotlib.pyplot`模块,并读取英文文本文件(如txt或html):
```python
from wordcloud import WordCloud
import matplotlib.pyplot as plt
with open('your_text_file.txt', 'r', encoding='utf-8') as file:
text = file.read()
```
3. 创建词云对象:使用`WordCloud`类,设置相应的参数,比如背景颜色、最大词数、字体路径等:
```python
wordcloud = WordCloud(font_path='path_to_your_font.ttf', width=800, height=600, background_color='white')
```
4. 计算词频并绘制词云:
```python
wordcloud.generate(text)
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.show()
```
5. 可选操作:还可以保存图片到本地:
```python
plt.savefig('english_wordcloud.png')
```
相关问题:
1. 在Python中,如何设置词云的颜色和大小?
2. 如何处理非英文字符或停用词在生成词云中的影响?
3. 如何调整词云的布局和样式?
阅读全文
相关推荐
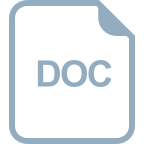
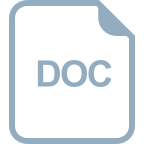
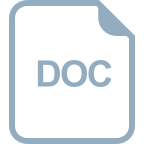


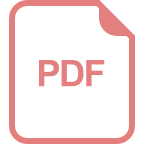
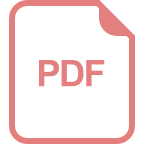
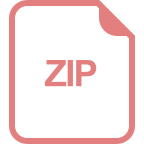

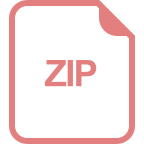
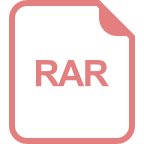
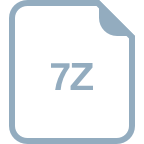
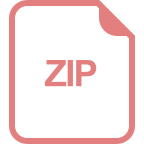
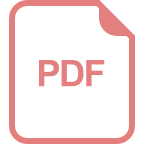


