用P、V操作实现哲学家进餐问题的第2种解法
时间: 2024-05-24 20:13:26 浏览: 85
可以使用信号量(Semaphore)实现哲学家进餐问题的第2种解法。具体实现方式是,每个哲学家都有一个信号量,表示他的左右两边的餐叉是否可用。当哲学家想要进餐时,他必须先获取他左右两边的餐叉,如果餐叉都可用,则他获取到信号量并开始进餐,否则他会等待直到餐叉可用。当他吃完后,他会释放他左右两边的餐叉的信号量,使得其他哲学家可以使用这些餐叉。
相关问题
用java实现实现哲学家进餐问题
哲学家进餐问题是一个经典的同步问题,主要涉及到多个进程(哲学家)之间的资源竞争问题。在这个问题中,每个哲学家都需要交替地进行思考和进餐,但是他们共享一些资源,如餐具等,因此需要协调它们的行为以避免死锁和饥饿等问题。
下面是使用 Java 实现哲学家进餐问题的示例代码:
```java
import java.util.concurrent.Semaphore;
public class Philosopher implements Runnable {
private final int id;
private final Semaphore leftFork;
private final Semaphore rightFork;
public Philosopher(int id, Semaphore leftFork, Semaphore rightFork) {
this.id = id;
this.leftFork = leftFork;
this.rightFork = rightFork;
}
@Override
public void run() {
try {
while (true) {
think();
leftFork.acquire();
rightFork.acquire();
eat();
rightFork.release();
leftFork.release();
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
return;
}
}
private void think() throws InterruptedException {
System.out.println("Philosopher " + id + " is thinking");
Thread.sleep((long) (Math.random() * 5000));
}
private void eat() throws InterruptedException {
System.out.println("Philosopher " + id + " is eating");
Thread.sleep((long) (Math.random() * 5000));
}
}
public class DiningPhilosophers {
private static final int NUM_PHILOSOPHERS = 5;
public static void main(String[] args) {
Semaphore[] forks = new Semaphore[NUM_PHILOSOPHERS];
for (int i = 0; i < NUM_PHILOSOPHERS; i++) {
forks[i] = new Semaphore(1);
}
Philosopher[] philosophers = new Philosopher[NUM_PHILOSOPHERS];
for (int i = 0; i < NUM_PHILOSOPHERS; i++) {
philosophers[i] = new Philosopher(i, forks[i], forks[(i + 1) % NUM_PHILOSOPHERS]);
new Thread(philosophers[i]).start();
}
}
}
```
在这个示例代码中,我们创建了一个 `Philosopher` 类和一个 `DiningPhilosophers` 类,其中 `Philosopher` 类实现了哲学家的行为,而 `DiningPhilosophers` 类则负责创建和启动哲学家线程。
在 `Philosopher` 类中,我们使用了两个信号量 `leftFork` 和 `rightFork` 表示哲学家左边和右边的餐叉。在 `run` 方法中,哲学家先思考一段时间,然后尝试获取左右餐叉的信号量。如果成功获取了两个餐叉的信号量,哲学家就可以进餐。最后,哲学家释放左右餐叉的信号量,以便其他哲学家可以使用它们。
在 `DiningPhilosophers` 类中,我们首先创建了一个 `Semaphore` 数组 `forks`,其中每个元素都表示一个餐叉。然后,我们创建了五个 `Philosopher` 对象,并将它们的左右餐叉设置为相邻的两个 `Semaphore` 对象。最后,我们创建了五个线程,每个线程都对应一个哲学家,并启动它们。当程序运行时,每个哲学家会交替进行思考和进餐,直到程序被中断或终止。
给出操作系统中利用记录型信号量解决哲学家进餐问题中的P,V操作实现的伪代码
在操作系统中,哲学家进餐问题是经典的一道并发控制问题,它涉及五个哲学家围坐在一起共享面包和橄榄,并通过互斥地获取左右筷子来进行思考和进食。这里我们使用记录型信号量(Semaphore with resources)来解决这个问题。
首先,我们需要两个类型的信号量,一个用于表示筷子的数量(例如,每只筷子一个信号量),另一个表示当前可用于使用的餐桌。假设我们用`philosopher_semaphores`存储筷子信号量数组,`table_semaphore`存储餐桌信号量。
**伪代码**:
```python
// 初始化信号量值
初始化PhilosopherSemaphores(5) // 筷子数量为5
初始化TableSemaphore(1) // 只有一张餐桌
function philosopher(id):
while True:
// P1: 尝试获取左筷子
acquire(philosopher_semaphores[id])
// P2: 获取右筷子
acquire(philosopher_semaphores[(id + 1) % 5]) // 循环索引防止数组溢出
// P3: 享用食物 (思考)
think()
// V1: 放回右筷子
release(philosopher_semaphores[(id + 1) % 5])
// V2: 放回左筷子
release(philosopher_semaphores[id])
// P4: 请求餐桌
acquire(table_semaphore)
// 如果所有哲学家都在等待,则唤醒一个
if all_philosophers_waiting():
wake_up_random_philosopher()
function think():
// 假设哲学家思考的时间随机
delay(random_time())
function all_philosophers_waiting():
return table_semaphore.value == 0 and
all(philosopher_semaphores[i].value == 0 for i in range(5))
function wake_up_random_philosopher():
// 随机选择一个未占用餐桌的哲学家唤醒
target_id = random.choice([i for i in range(5) if philosopher_semaphores[i].value > 0])
philosopher_semaphores[target_id].decrement() // 唤醒并释放餐桌
```
阅读全文
相关推荐
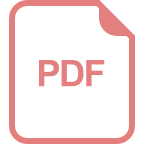
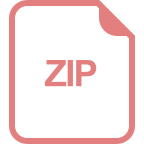
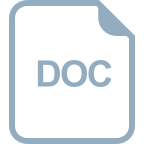
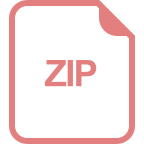
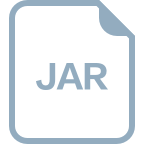
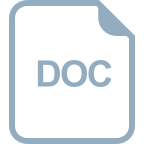



