DES算法 C语言
时间: 2023-11-25 07:02:13 浏览: 33
DES算法(Data Encryption Standard)是一种对称加密算法,它是一种分组密码,以64位为分组对数据进行加密。下面是一个使用C语言实现DES算法的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/des.h>
void des_encrypt(char *key, char *input, char *output)
{
DES_cblock key2;
DES_key_schedule schedule;
memcpy(key2, key, 8);
DES_set_key_unchecked(&key2, &schedule);
DES_ecb_encrypt((DES_cblock *)input, (DES_cblock *)output, &schedule, DES_ENCRYPT);
}
void des_decrypt(char *key, char *input, char *output)
{
DES_cblock key2;
DES_key_schedule schedule;
memcpy(key2, key, 8);
DES_set_key_unchecked(&key2, &schedule);
DES_ecb_encrypt((DES_cblock *)input, (DES_cblock *)output, &schedule, DES_DECRYPT);
}
int main()
{
char key[] = "12345678"; // 密钥
char input[] = "hello world"; // 明文
char output[64]; // 密文
des_encrypt(key, input, output);
printf("Encrypted: %s\n", output);
des_decrypt(key, output, input);
printf("Decrypted: %s\n", input);
return 0;
}
```
该示例使用了OpenSSL库中的DES函数,其中`des_encrypt`函数用于加密,`des_decrypt`函数用于解密。输入的密钥和明文都是字符数组,输出的密文也是字符数组。在加密和解密的过程中,都使用了相同的密钥。
相关推荐
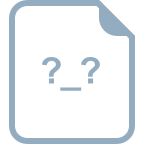














