yolo v7层面提高map值的代码
时间: 2023-08-07 10:02:28 浏览: 234
要提高YOLOv7的mAP值,可以考虑以下几个方面:
1. 使用更大的训练数据集,包含更多的类别和更多的样本。可以从公开数据集中获取更多的数据,或者使用数据增强技术扩充现有数据集。
2. 调整网络结构和超参数,例如增加网络的深度或宽度,调整学习率和优化器等。
3. 使用更好的模型结构,例如使用更先进的检测模型如EfficientDet或CenterNet。
4. 加强训练过程,例如使用更好的损失函数,增加正则化项等。
以下是一个简单的代码示例,展示如何在YOLOv7中使用CutMix数据增强方法来提高mAP值:
```
# 使用CutMix数据增强方法
def cutmix(batch, alpha):
lam = np.random.beta(alpha, alpha)
rand_index = torch.randperm(batch.size()[0]).cuda()
target_a = batch[:, :, :, :]
target_b = batch[rand_index, :, :, :]
height = batch.size()[2]
width = batch.size()[3]
bbx1, bby1, bbx2, bby2 = rand_bbox(width, height, lam)
batch[:, :, bbx1:bbx2, bby1:bby2] = target_b[:, :, bbx1:bbx2, bby1:bby2]
lam = 1 - ((bbx2 - bbx1) * (bby2 - bby1) / (width * height))
return batch, target_a, target_b, lam
# 计算随机裁剪的bbox坐标
def rand_bbox(width, height, lam):
cut_w = np.int(width * lam)
cut_h = np.int(height * lam)
# uniform
cx = np.random.randint(width)
cy = np.random.randint(height)
bbx1 = np.clip(cx - cut_w // 2, 0, width)
bby1 = np.clip(cy - cut_h // 2, 0, height)
bbx2 = np.clip(cx + cut_w // 2, 0, width)
bby2 = np.clip(cy + cut_h // 2, 0, height)
return bbx1, bby1, bbx2, bby2
# 训练模型
for epoch in range(num_epochs):
for i, (images, labels) in enumerate(train_loader):
images = images.to(device)
labels = labels.to(device)
# 使用CutMix数据增强方法
images, target_a, target_b, lam = cutmix(images, alpha)
# 前向传播
outputs = model(images)
loss = criterion(outputs, target_a) * lam + criterion(outputs, target_b) * (1. - lam)
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 每隔一定的步数输出信息
if (i + 1) % print_freq == 0:
print('Epoch [{}/{}], Step [{}/{}], Loss: {:.4f}'
.format(epoch + 1, num_epochs, i + 1, total_step, loss.item()))
# 在验证集上进行评估
with torch.no_grad():
model.eval()
for images, labels in val_loader:
images = images.to(device)
labels = labels.to(device)
outputs = model(images)
val_loss = criterion(outputs, labels)
val_loss += val_loss.item()
# 计算mAP值
ap, map = compute_mAP(model, val_loader, device)
print('Validation Loss: {:.4f}, mAP: {:.4f}'.format(val_loss, map))
model.train()
```
上述代码示例中,CutMix数据增强方法可以在训练过程中随机裁剪图像,并将裁剪后的图像片段与其他图像进行混合,从而增加了训练数据的多样性。在计算损失函数时,需要根据裁剪的比例计算每个样本的权重。在验证集上,可以使用compute_mAP函数计算模型在验证集上的mAP值。
阅读全文
相关推荐
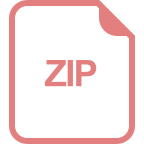
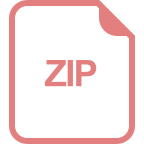
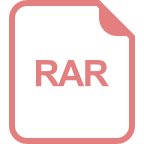















