单链表的增删改查代码
时间: 2024-09-25 14:00:46 浏览: 29
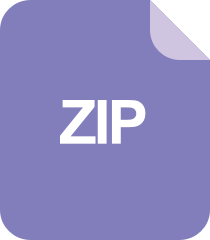
Java单链表增删改查的实现
单链表的基本增删改查操作可以用不同的编程语言实现,这里以Python为例:
1. **创建/插入节点**:
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
def append(head, data):
if not head:
return Node(data)
curr = head
while curr.next:
curr = curr.next
curr.next = Node(data)
return head
```
2. **查找/删除节点**:
```python
def search_and_delete(head, target):
if not head:
return None
if head.data == target:
return head.next
curr = head
while curr.next and curr.next.data != target:
curr = curr.next
if curr.next is None:
return head
else:
curr.next = curr.next.next
return head
```
3. **修改节点**:
```python
def update_node_value(head, key, new_data):
curr = head
while curr:
if curr.data == key:
curr.data = new_data
break
curr = curr.next
return head
```
4. **查询节点个数**:
```python
def count_nodes(head):
count = 0
curr = head
while curr:
count += 1
curr = curr.next
return count
```
阅读全文
相关推荐
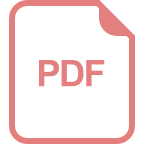
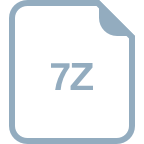
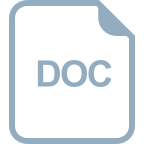




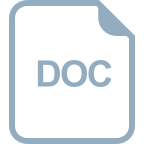








