寻找一个海洋图像,统计这幅图像的直方图。用python代码对图像进行截断和量化
时间: 2024-04-26 11:24:50 浏览: 85
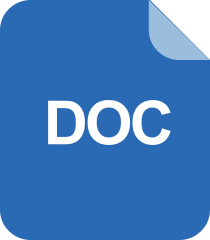
基于直方图的图像检索

以下是一个使用 Python 和 OpenCV 库对海洋图像进行直方图统计、截断和量化的示例代码:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 读取海洋图像
img = cv2.imread('ocean.jpg', cv2.IMREAD_GRAYSCALE)
# 统计图像直方图
hist, bins = np.histogram(img.flatten(), 256, [0, 256])
# 绘制直方图
plt.hist(img.flatten(), 256, [0, 256])
plt.title('Histogram')
plt.xlabel('Gray Level')
plt.ylabel('Pixel Count')
plt.show()
# 对图像进行截断
img_trunc = np.clip(img, 100, 200)
# 统计截断后图像的直方图
hist_trunc, bins_trunc = np.histogram(img_trunc.flatten(), 256, [0, 256])
# 绘制截断后图像的直方图
plt.hist(img_trunc.flatten(), 256, [0, 256])
plt.title('Truncated Histogram')
plt.xlabel('Gray Level')
plt.ylabel('Pixel Count')
plt.show()
# 对图像进行量化
img_quant = np.round(img / 32) * 32
# 统计量化后图像的直方图
hist_quant, bins_quant = np.histogram(img_quant.flatten(), 256, [0, 256])
# 绘制量化后图像的直方图
plt.hist(img_quant.flatten(), 256, [0, 256])
plt.title('Quantized Histogram')
plt.xlabel('Gray Level')
plt.ylabel('Pixel Count')
plt.show()
```
这段代码假设您已经准备了一幅名为 `ocean.jpg` 的海洋图像。代码首先使用 OpenCV 库的 `imread()` 函数读取该图像,并将其转换为灰度图像。
然后,使用 NumPy 库的 `histogram()` 函数计算图像的直方图,并使用 Matplotlib 库的 `hist()` 函数绘制直方图。
接下来,代码使用 NumPy 库的 `clip()` 函数对图像进行截断。该函数将小于 100 和大于 200 的像素值分别截断为 100 和 200,并将其余像素值保持不变。然后,再次使用 `histogram()` 和 `hist()` 函数计算和绘制截断后图像的直方图。
最后,代码对图像进行量化。该过程将每个像素值四舍五入到最接近的 32 的倍数,并将其作为新的像素值。然后,再次使用 `histogram()` 和 `hist()` 函数计算和绘制量化后图像的直方图。
您可以根据需要修改参数和细节,以适应不同的应用场景。
阅读全文
相关推荐

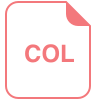
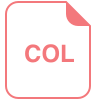
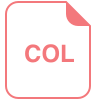
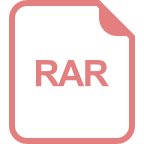
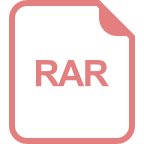
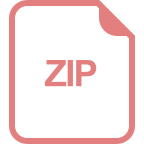
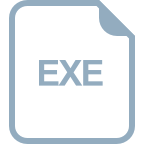
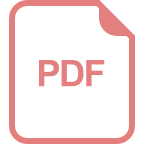