def days_of_month(date_str): """根据输入的年月日,返回该月的天数"""
时间: 2023-10-22 12:20:28 浏览: 151
以下是一个实现这个功能的代码:
```
import datetime
def days_of_month(date_str):
year, month, day = map(int, date_str.split('-'))
days_in_month = (datetime.date(year, month+1, 1) - datetime.date(year, month, 1)).days
return days_in_month
```
这个函数接收一个形如"YYYY-MM-DD"的日期字符串作为输入,然后解析出年、月、日三个部分。接着,使用Python标准库中的`datetime`模块计算出下一个月的第一天和这个月的第一天之间的天数差,即为该月的天数。最后,返回这个天数即可。
相关问题
任务描述 输入一个8位数表示的年月日,读出月份数字并输出该月有多少天。 输入格式 输入一个8位的表示年月日的字符串 输出格式 该月的天数 示例 输入: 20000219 输出: 29
这是一个编程任务,要求设计一个程序来解析输入的8位字符串(如 "20000219"),其中前四位代表年份,中间两位代表月份,最后两位代表日期。然后根据给定的年份和月份计算出对应的月份数量。例如,2月份在闰年(如2000年)有29天,在平年则有28天。此任务通常涉及到对闰年规则的理解和日期算法的实现。
以下是Python的一个简单示例代码片段:
```python
def days_of_month(date_str):
year, month, _ = map(int, date_str[:4], date_str[4:6], date_str[6:])
days_in_month = [31, 28 if not is_leap(year) else 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
return days_in_month[month - 1]
def is_leap(year):
return year % 4 == 0 and (year % 100 != 0 or year % 400 == 0)
date_in = input().strip() # 获取用户输入的年月日字符串
print(days_of_month(date_in))
```
在这个代码中,`days_of_month` 函数首先分割输入字符串得到年、月、日,然后从预设的列表中取出对应月份的天数。`is_leap` 函数用于检查年份是否为闰年。最后,将用户输入的年月日传递给 `days_of_month` 函数,输出结果。
任务描述 输入一个8位数表示的年月日,读出月份数字并输出该月有多少天。 输入格式 输入一个8位的表示年月日的字符串 输出格式 该月的天数 示例 输入: 20000219 输出: 29 用Python写代码
你可以使用以下Python代码来实现该任务:
```python
def get_month_days(date_str):
year = int(date_str[:4])
month = int(date_str[4:6])
# 判断是否为闰年
if year % 4 == 0 and year % 100 != 0 or year % 400 == 0:
leap_year = True
else:
leap_year = False
# 判断每个月的天数
if month in [1, 3, 5, 7, 8, 10, 12]:
days = 31
elif month in [4, 6, 9, 11]:
days = 30
elif month == 2:
if leap_year:
days = 29
else:
days = 28
else:
days = -1
return days
date_str = input("请输入一个8位的表示年月日的字符串:")
month_days = get_month_days(date_str)
print(month_days)
```
请注意,上述代码中的输入部分使用了`input()`函数,可以通过用户输入来获取年月日的字符串。输出部分使用了`print()`函数来打印该月的天数。
阅读全文
相关推荐
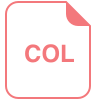
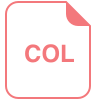
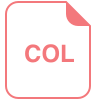
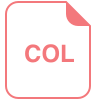









