帮我写c语言代码能够体现:创建线程并显示多线程协同工作过程
时间: 2024-12-17 17:43:55 浏览: 0
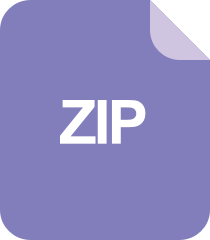
C语言程序设计大作业:学生成绩管理系统代码(Qt).zip
在 C 语言中,我们可以利用 POSIX 线程库(pthread.h)来创建和管理线程。下面是一个简单的示例,展示了如何创建两个线程,并通过共享变量同步他们的输出:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
// 全局共享变量
int shared_count = 0;
// 输出计数函数
void* count_thread(void* arg) {
int thread_id = (int)arg;
printf("Thread %d started.\n", thread_id);
for (int i = 0; i < 5; ++i) {
// 模拟一些计算
sleep(1); // 让线程暂停一下,模拟耗时操作
shared_count++;
printf("Thread %d incremented shared count to %d\n", thread_id, shared_count);
}
printf("Thread %d finished.\n", thread_id);
pthread_exit(NULL);
}
int main() {
pthread_t thread1, thread2; // 创建两个线程
int thread_ids[] = {1, 2}; // 存储线程ID
// 创建并启动线程
for (int i = 0; i < 2; ++i) {
if (pthread_create(&thread_ids[i], NULL, count_thread, &thread_ids[i]) != 0) {
perror("Error creating thread");
exit(EXIT_FAILURE);
}
}
// 等待所有线程完成
for (int i = 0; i < 2; ++i) {
pthread_join(thread_ids[i], NULL);
}
printf("Shared count after all threads completed: %d\n", shared_count);
return 0;
}
```
在这个例子中,`count_thread` 函数会被两个线程并发执行,它们都会增加全局 `shared_count` 变量的值。通过 `sleep(1)`,我们可以看到线程之间的协同工作——尽管它们不是同步执行的,但是结果保证了共享变量的更新顺序。
阅读全文
相关推荐
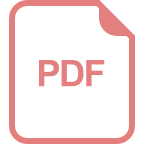
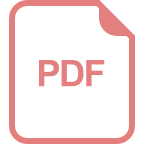
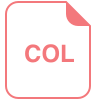

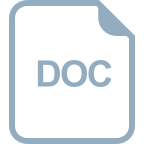
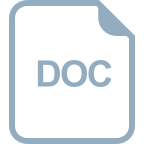
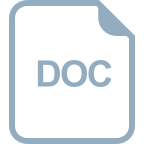
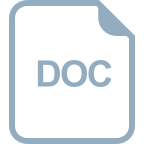
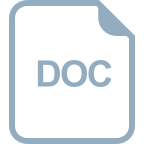
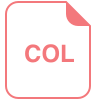
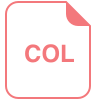
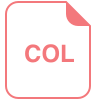
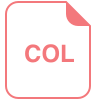
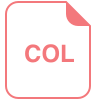
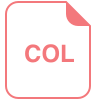
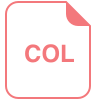
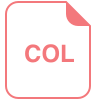
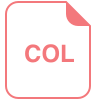