qt中的qtimer怎么加到std::thread中去
时间: 2023-06-23 10:51:07 浏览: 169
在Qt中,QTimer是基于事件循环机制实现的,不能直接添加到std::thread线程中。如果你需要在std::thread线程中使用定时器,可以通过以下步骤来实现:
1. 创建一个单独的QObject对象,用于管理定时器并将其移动到std::thread线程中。
2. 在该QObject对象中创建一个QTimer对象,并设置定时器的时间间隔和处理程序。
3. 将该QObject对象移动到std::thread线程中。
4. 在std::thread线程中启动一个事件循环,以便能够处理定时器事件。
以下是一个示例代码:
```
#include <QObject>
#include <QTimer>
#include <thread>
class MyTimer : public QObject
{
Q_OBJECT
public:
MyTimer(int interval, QObject *parent = nullptr) : QObject(parent)
{
m_timer = new QTimer(this);
m_timer->setInterval(interval);
connect(m_timer, &QTimer::timeout, this, &MyTimer::onTimeout);
}
void start()
{
moveToThread(&m_thread);
m_thread.start();
m_timer->start();
}
signals:
void timeout();
private slots:
void onTimeout()
{
emit timeout();
}
private:
QTimer *m_timer;
QThread m_thread;
};
int main()
{
MyTimer timer(1000);
std::thread thread([&](){
QCoreApplication app(argc, argv);
timer.start();
app.exec();
});
QObject::connect(&timer, &MyTimer::timeout, [](){
qDebug() << "Timer timeout";
});
thread.join();
return 0;
}
```
在这个示例代码中,我们创建了一个MyTimer类,在其中创建了一个QTimer对象,并将其移动到了一个单独的线程中。然后,在std::thread线程中创建了一个QCoreApplication对象,并启动了一个事件循环,以便能够处理定时器事件。最后,我们连接了MyTimer的timeout信号到槽函数中,以便能够在定时器超时时执行一些操作。
阅读全文
相关推荐
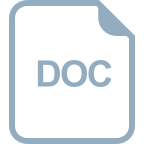
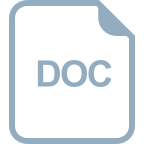
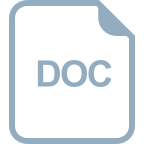




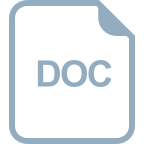
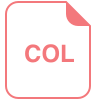
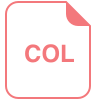
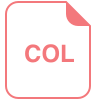
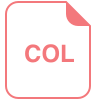
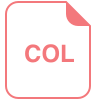
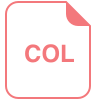
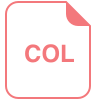



