c编程实现strlen函数的功能
时间: 2024-01-01 14:04:50 浏览: 55
strlen函数可以得出字符串的长度,下面是一个C语言实现的strlen函数:
```c
#include <stdio.h>
#include <string.h>
int my_strlen(char *str){
int len = 0;
while(*str != '\0'){
len++;
str++;
}
return len;
}
int main(){
char str[] = "hello, world!";
int len = my_strlen(str);
printf("The length of the string is: %d\n", len);
return 0;
}
```
这个函数通过循环遍历字符串,每遍历到一个字符就将计数器`len`加1,最后返回`len`的值。
相关问题
C语言编程实现strlen()函数功能
下面是 C 语言编写的 strlen() 函数代码:
```c
#include <stdio.h>
int strlen(char *str) {
int count = 0;
while (*str != '\0') {
count++;
str++;
}
return count;
}
int main() {
char str[] = "Hello World";
printf("The length of the string is %d", strlen(str));
return 0;
}
```
该函数使用指针遍历字符串,每次遍历时将计数器加一,直到遍历到字符串末尾的空字符为止。在主函数中,我们定义一个字符串常量并通过函数计算其长度并输出。
编程实现strlen函数的功能
可以使用循环遍历字符串中的每个字符,计算字符个数直到遇到字符串结尾符号 '\0'。以下是使用 C 语言实现 strlen 函数的示例代码:
```
#include <stdio.h>
int strlen(const char* str) {
int len = 0;
while (*str != '\0') {
len++;
str++;
}
return len;
}
int main() {
char* str = "hello world";
int len = strlen(str);
printf("The length of the string is: %d\n", len);
return 0;
}
```
相关推荐
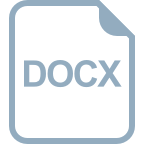












