用c语言编写一道程序 一次比赛中有n个评委打分,去掉一个最高分,一个最低分,求最后的平均得分
时间: 2024-12-22 09:18:35 浏览: 5
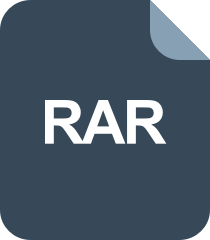
c#编写的打分程序(去掉最高分、最低分)

在C语言中,你可以通过动态数组来存储每个评委的分数,并计算最终平均分。这里是一个简单的示例程序:
```c
#include <stdio.h>
#define MAX_JUDGES 10 // 定义最大评委数
double calculate_average(int scores[], int n) {
if (n < 2) { // 如果评委数少于2,无法去除最高和最低分
printf("Error: At least two judges are needed.\n");
return -1; // 返回错误标志
}
// 排序评委得分
qsort(scores, n, sizeof(int), compare_scores);
// 去除最高分和最低分
double sum = scores[0]; // 最低分开始累加
for (int i = 1; i < n - 1; i++) { // 只考虑中间 n-2 个得分
sum += scores[i];
}
// 计算并返回平均分
return sum / (n - 2);
}
// 比较函数用于qsort排序
int compare_scores(const void *a, const void *b) {
return (*(const int*)a) - (*(const int*)b);
}
int main() {
int num_judges;
printf("Enter the number of judges: ");
scanf("%d", &num_judges);
int scores[MAX_JUDGES];
for (int i = 0; i < num_judges; i++) {
printf("Judge %d's score: ", i + 1);
scanf("%d", &scores[i]);
}
double average_score = calculate_average(scores, num_judges);
if (average_score != -1) {
printf("The average score after removing highest and lowest is %.2lf.\n", average_score);
}
return 0;
}
```
在这个程序中,用户首先输入评委人数和各自得分,然后程序会计算并打印出去除非最高和最低分后的平均分。
阅读全文
相关推荐
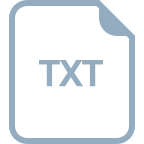
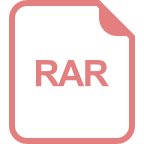















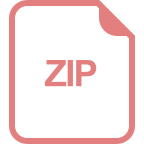