现在有一张用户登录表 (user_login_log),有userid,和login_time字段,用户每登录一次产生一条记录(用户一天多次登录,产生多条记录),现在查询出一段时间内,连续登录三天的用...
时间: 2024-03-16 21:45:17 浏览: 15
好的,针对你的问题,可以使用以下 SQL 语句来查询:
```
SELECT DISTINCT userid
FROM user_login_log
WHERE login_time >= '2022-01-01'
AND login_time < '2022-01-10'
GROUP BY userid, DATE(login_time)
HAVING COUNT(DISTINCT DATE(login_time)) >= 3;
```
其中,`'2022-01-01'` 和 `'2022-01-10'` 分别为查询的起止时间,可以根据实际需求进行修改。
这个 SQL 语句的作用是先根据用户 ID 和登录日期进行分组,统计每个用户在查询时间范围内登录的天数,然后筛选出登录天数大于等于 3 的用户 ID。最后使用 `DISTINCT` 关键字去重,确保每个用户只出现一次在结果集中。
希望这个回答对你有帮助,如果有任何疑问,欢迎继续提问!
相关推荐
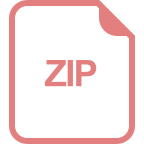
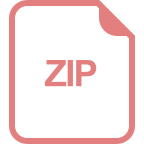
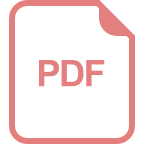
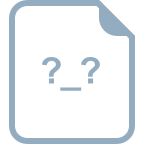
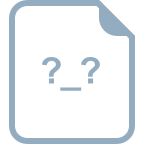