java多线程监听串口
时间: 2023-09-14 13:14:23 浏览: 191
在Java中监听串口可以使用Java Comm API。下面是一个简单的示例代码,演示如何使用Java Comm API在多线程中监听串口:
```java
import gnu.io.CommPortIdentifier;
import gnu.io.SerialPort;
import gnu.io.SerialPortEvent;
import gnu.io.SerialPortEventListener;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.util.Enumeration;
public class SerialCommunication implements Runnable, SerialPortEventListener {
private static final int TIME_OUT = 2000; //等待超时时间
private static final int BAUD_RATE = 9600; //波特率
private CommPortIdentifier portId;
private SerialPort serialPort;
private BufferedReader input;
private OutputStream output;
private boolean isRunning = false;
public static void main(String[] args) {
SerialCommunication communication = new SerialCommunication();
communication.start();
}
public void start() {
Thread thread = new Thread(this);
thread.start();
}
public void run() {
try {
//获取串口ID
Enumeration portEnum = CommPortIdentifier.getPortIdentifiers();
while (portEnum.hasMoreElements()) {
CommPortIdentifier currentPortId = (CommPortIdentifier) portEnum.nextElement();
if (currentPortId.getPortType() == CommPortIdentifier.PORT_SERIAL) {
portId = currentPortId;
break;
}
}
if (portId == null) {
System.out.println("没有发现串口");
return;
}
//打开串口并设置参数
serialPort = (SerialPort) portId.open(this.getClass().getName(), TIME_OUT);
serialPort.setSerialPortParams(BAUD_RATE, SerialPort.DATABITS_8, SerialPort.STOPBITS_1, SerialPort.PARITY_NONE);
//获取输入输出流
input = new BufferedReader(new InputStreamReader(serialPort.getInputStream()));
output = serialPort.getOutputStream();
//添加串口事件监听器
serialPort.addEventListener(this);
serialPort.notifyOnDataAvailable(true);
System.out.println("串口已打开,开始监听...");
isRunning = true;
while (isRunning) {
Thread.sleep(1000);
}
//关闭串口
serialPort.removeEventListener();
serialPort.close();
input.close();
output.close();
System.out.println("串口已关闭");
} catch (Exception ex) {
System.out.println("打开串口失败:" + ex.getMessage());
}
}
public void stop() {
isRunning = false;
}
public void serialEvent(SerialPortEvent event) {
if (event.getEventType() == SerialPortEvent.DATA_AVAILABLE) {
try {
String inputLine = input.readLine();
System.out.println("接收到数据:" + inputLine);
} catch (Exception ex) {
System.out.println("读取串口数据失败:" + ex.getMessage());
}
}
}
}
```
该示例代码中,首先使用`CommPortIdentifier`类获取所有串口的ID,然后找到需要监听的串口的ID。接着打开串口并设置参数,获取输入输出流,并添加串口事件监听器。在`serialEvent`方法中,可以处理从串口接收到的数据。`start`方法创建一个新的线程,该线程调用`run`方法开始监听串口。`stop`方法可以停止监听。
使用多线程的好处是可以在主线程中执行其他任务,而不会阻塞串口的监听。当然,在处理串口数据时也可以使用多线程。
阅读全文
相关推荐



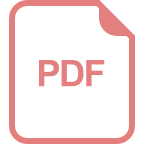
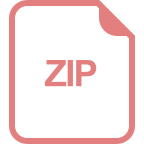
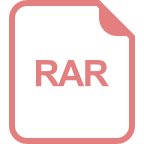
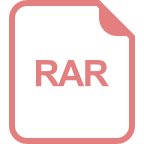
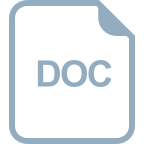
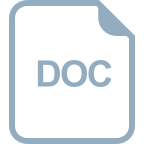
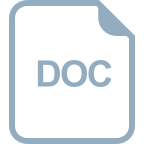
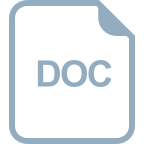
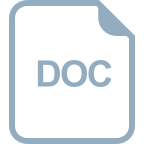

