创建一个名为 Lab4_ex3 的新 java 类,并创建一个数组名称为 grade、数组大小为 5 的数组,提示用户通过使用 scanner 类将数据输入到数组中来输入他们的测试分数。使用 for 循环通过添加和更新名为 “Lab4.txt” 的相同文件来打印数组测试的所有元素
时间: 2024-10-21 16:09:42 浏览: 21
在Java中,你可以按照以下步骤创建名为`Lab4_ex3`的新类,并实现提示用户输入测试分数以及保存和读取分数到文本文件的功能:
```java
import java.io.*;
import java.util.Scanner;
public class Lab4_ex3 {
private int[] grade; // 定义grade数组
private static final int SIZE = 5; // 设置数组大小
public Lab4_ex3() {
grade = new int[SIZE]; // 初始化数组
Scanner input = new Scanner(System.in);
// 提示用户输入测试分数并存储到数组
System.out.println("请输入学生的测试分数,共" + SIZE + "项:");
for (int i = 0; i < SIZE; i++) {
grade[i] = input.nextInt();
}
saveToFile(); // 存储分数到文件
}
private void saveToFile() {
try (BufferedWriter writer = new BufferedWriter(new FileWriter("Lab4.txt"))) {
for (int score : grade) {
writer.write(score + "\n");
}
} catch (IOException e) {
System.err.println("Error writing to file: " + e.getMessage());
}
}
public static void main(String[] args) {
new Lab4_ex3();
// 为了在main方法中查看内容,可以在这里读取并打印文件内容
try (BufferedReader reader = new BufferedReader(new FileReader("Lab4.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line); // 打印文件里的每一行
}
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
}
}
```
在这个例子中,我们在`Lab4_ex3`类中创建了一个整数数组`grade`,然后使用Scanner从控制台获取用户输入的数据。接着,我们将这些分数保存到名为"Lab4.txt"的文件中。主函数`main`里展示了如何读取这个文件的内容。
阅读全文
相关推荐
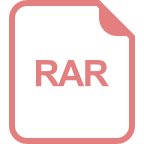
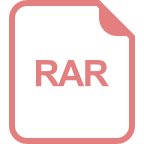
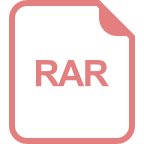
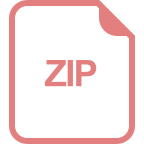
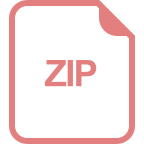
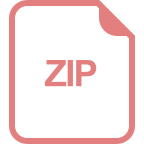
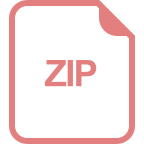
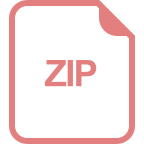
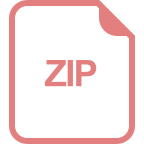
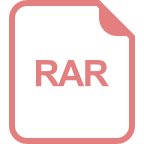
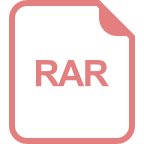
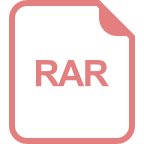
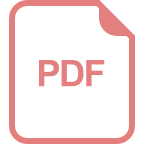
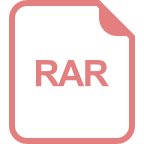
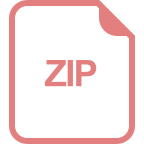
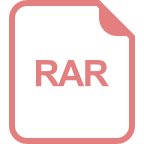
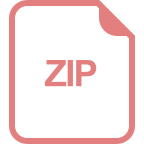
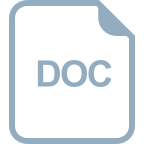
