java编写一个图书管理系统
时间: 2024-05-28 20:05:10 浏览: 107
Java是一种面向对象的编程语言,它可以用于开发各种类型的应用程序,包括图书管理系统。下面是一个简单的Java图书管理系统的实现方式:
1. 首先,需要定义一个Book类,该类包含书籍的基本信息,如书名、作者、出版社、ISBN号等。可以使用以下代码定义Book类:
```
public class Book {
private String title;
private String author;
private String publisher;
private String isbn;
public Book(String title, String author, String publisher, String isbn) {
this.title = title;
this.author = author;
this.publisher = publisher;
this.isbn = isbn;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getPublisher() {
return publisher;
}
public String getIsbn() {
return isbn;
}
}
```
2. 接下来,需要定义一个BookManager类,该类包含对图书进行管理的方法,如添加图书、删除图书、查找图书等。可以使用以下代码定义BookManager类:
```
import java.util.ArrayList;
import java.util.List;
public class BookManager {
private List<Book> books;
public BookManager() {
books = new ArrayList<>();
}
public void addBook(Book book) {
books.add(book);
}
public void removeBook(Book book) {
books.remove(book);
}
public Book findBookByTitle(String title) {
for (Book book : books) {
if (book.getTitle().equals(title)) {
return book;
}
}
return null;
}
public Book findBookByAuthor(String author) {
for (Book book : books) {
if (book.getAuthor().equals(author)) {
return book;
}
}
return null;
}
public Book findBookByIsbn(String isbn) {
for (Book book : books) {
if (book.getIsbn().equals(isbn)) {
return book;
}
}
return null;
}
}
```
3. 最后,可以编写一个简单的控制台程序来测试图书管理系统。可以使用以下代码实现:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
BookManager manager = new BookManager();
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("请选择操作:");
System.out.println("1. 添加图书");
System.out.println("2. 删除图书");
System.out.println("3. 查找图书");
System.out.println("4. 退出");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
System.out.println("请输入书名:");
String title = scanner.nextLine();
System.out.println("请输入作者:");
String author = scanner.nextLine();
System.out.println("请输入出版社:");
String publisher = scanner.nextLine();
System.out.println("请输入ISBN号:");
String isbn = scanner.nextLine();
Book book = new Book(title, author, publisher, isbn);
manager.addBook(book);
System.out.println("添加成功!");
break;
case 2:
System.out.println("请输入书名:");
title = scanner.nextLine();
book = manager.findBookByTitle(title);
if (book != null) {
manager.removeBook(book);
System.out.println("删除成功!");
} else {
System.out.println("未找到该书!");
}
break;
case 3:
System.out.println("请选择查找方式:");
System.out.println("1. 按书名查找");
System.out.println("2. 按作者查找");
System.out.println("3. 按ISBN号查找");
int searchChoice = scanner.nextInt();
scanner.nextLine();
switch (searchChoice) {
case 1:
System.out.println("请输入书名:");
title = scanner.nextLine();
book = manager.findBookByTitle(title);
if (book != null) {
System.out.println("书名:" + book.getTitle());
System.out.println("作者:" + book.getAuthor());
System.out.println("出版社:" + book.getPublisher());
System.out.println("ISBN号:" + book.getIsbn());
} else {
System.out.println("未找到该书!");
}
break;
case 2:
System.out.println("请输入作者:");
author = scanner.nextLine();
book = manager.findBookByAuthor(author);
if (book != null) {
System.out.println("书名:" + book.getTitle());
System.out.println("作者:" +
阅读全文
相关推荐
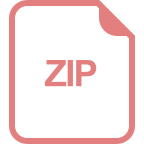
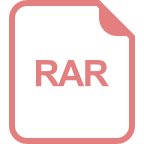
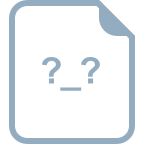
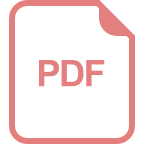
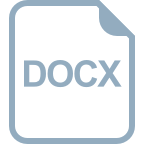
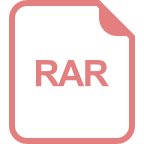
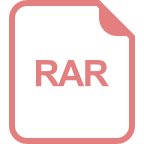
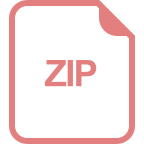
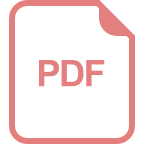
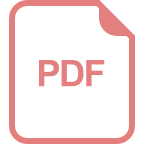
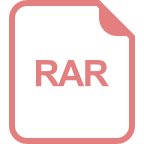
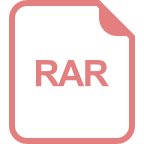
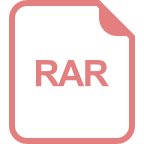
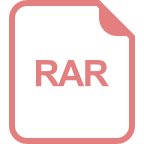