public void Move() { int stt = sun; scoreText.text = stt.ToString(); if (Input.GetKey(KeyCode.W)) { direction = 0; this.gameObject.GetComponent<SpriteRenderer>().sprite = prota[2]; this.gameObject.transform.Translate(Vector3.up * speed * Time.deltaTime); } else if (Input.GetKey(KeyCode.S)) { this.gameObject.GetComponent<SpriteRenderer>().sprite = prota[0]; direction = 2; this.gameObject.transform.Translate(Vector3.down * speed * Time.deltaTime); } else if (Input.GetKey(KeyCode.D)) { direction = 3; this.gameObject.GetComponent<SpriteRenderer>().sprite = prota[1]; this.gameObject.transform.Translate(Vector3.right * speed * Time.deltaTime); } else if (Input.GetKey(KeyCode.A)) { direction = 1; this.gameObject.GetComponent<SpriteRenderer>().sprite = prota[3]; this.gameObject.transform.Translate(Vector3.left * speed * Time.deltaTime); } // 播放脚步声 if (Input.GetKey(KeyCode.W) || Input.GetKey(KeyCode.S) || Input.GetKey(KeyCode.A) || Input.GetKey(KeyCode.D)) { if (!audioSource.isPlaying) { audioSource.clip = footstepSound; audioSource.loop = true; audioSource.Play(); } } else { audioSource.Stop(); } // 攻击 if (Input.GetMouseButtonDown(0)) { // 处理鼠标左键点击事件 GameObject ins = Instantiate(bulletPrefab); Vector2 clickPosition = Camera.main.ScreenToWorldPoint(Input.mousePosition); ins.GetComponent<Blogs>().clickPosition = clickPosition; if (direction == 0) { ins.transform.position = new Vector3(this.gameObject.transform.position.x, this.gameObject.transform.position.y + 0.15f); } else if (direction == 2) { ins.transform.position = new Vector3(this.gameObject.transform.position.x, this.gameObject.transform.position.y - 0.15f); } else if (direction == 3) { ins.transform.position = new Vector3(this.gameObject.transform.position.x + 0.15f, this.gameObject.transform.position.y); } else if (direction == 1) { ins.transform.position = new Vector3(this.gameObject.transform.position.x - 0.15f, this.gameObject.transform.position.y); } } }public void OnCollisionEnter2D(Collision2D collision) { if(collision.gameObject.tag == "GoldCoin") { Destroy(collision.gameObject); sun = sun + 1; if (!audioSource.isPlaying) { audioSource.clip = shique; audioSource.loop = true; audioSource.Play(); } } else { audioSource.Stop(); } //判断是否遭受攻击 if (collision.gameObject.tag == "zhi") { Destroy(collision.gameObject); hp = hp - 1; } // 检测碰撞物体的标签 if (collision.gameObject.tag == "zhi2") { // 忽略该碰撞 Physics2D.IgnoreCollision(collision.collider, GetComponent<Collider2D>()); } }优化代码
时间: 2023-06-24 19:08:37 浏览: 165
1. 将变量和函数命名规范化,遵循驼峰式命名法。
2. 将常量和不变的数值提取出来,定义为常量或静态变量。
3. 合理利用 Unity 引擎提供的功能,如使用 GetComponent 获取组件、使用 ScreenToWorldPoint 将屏幕坐标转换为世界坐标等。
4. 使用 switch 语句代替 if-else 语句,可以提高代码可读性和效率。
5. 在需要处理多个碰撞标签时,可以使用 switch-case 语句代替多个 if-else 语句。
修改后的代码如下:
```C#
public class PlayerController : MonoBehaviour {
private const float Speed = 5f; // 移动速度
private const int MaxHp = 5; // 最大生命值
private const float BulletOffset = 0.15f; // 子弹偏移量
[SerializeField] private AudioClip footstepSound; // 脚步声音效
[SerializeField] private AudioClip shique; // 收集金币音效
[SerializeField] private GameObject bulletPrefab; // 子弹预制体
[SerializeField] private Sprite[] prota; // 精灵数组
[SerializeField] private Text scoreText; // 分数文本
private AudioSource audioSource; // 音频源
private int hp = MaxHp; // 当前生命值
private int score; // 得分
private int direction; // 移动方向
private void Start() {
audioSource = GetComponent<AudioSource>();
}
private void Update() {
Move();
Attack();
}
private void Move() {
scoreText.text = score.ToString();
Vector3 movement = Vector3.zero;
if (Input.GetKey(KeyCode.W)) {
direction = 0;
GetComponent<SpriteRenderer>().sprite = prota[2];
movement = Vector3.up;
} else if (Input.GetKey(KeyCode.S)) {
direction = 2;
GetComponent<SpriteRenderer>().sprite = prota[0];
movement = Vector3.down;
} else if (Input.GetKey(KeyCode.D)) {
direction = 3;
GetComponent<SpriteRenderer>().sprite = prota[1];
movement = Vector3.right;
} else if (Input.GetKey(KeyCode.A)) {
direction = 1;
GetComponent<SpriteRenderer>().sprite = prota[3];
movement = Vector3.left;
}
transform.Translate(movement * Speed * Time.deltaTime);
PlayFootstepSound(Input.GetKey(KeyCode.W) || Input.GetKey(KeyCode.S) || Input.GetKey(KeyCode.A) || Input.GetKey(KeyCode.D));
}
private void PlayFootstepSound(bool isMoving) {
if (isMoving && !audioSource.isPlaying) {
audioSource.clip = footstepSound;
audioSource.loop = true;
audioSource.Play();
} else if (!isMoving) {
audioSource.Stop();
}
}
private void Attack() {
if (Input.GetMouseButtonDown(0)) {
GameObject bullet = Instantiate(bulletPrefab);
Vector2 clickPosition = Camera.main.ScreenToWorldPoint(Input.mousePosition);
bullet.GetComponent<Bullet>().SetDirection(direction);
bullet.transform.position = transform.position + BulletOffset * (Vector3)clickPosition.normalized;
}
}
private void OnTriggerEnter2D(Collider2D other) {
switch (other.tag) {
case "GoldCoin":
CollectCoin(other.gameObject);
break;
case "zhi":
TakeDamage(1);
break;
}
}
private void OnTriggerStay2D(Collider2D other) {
if (other.tag == "zhi2") {
Physics2D.IgnoreCollision(other, GetComponent<Collider2D>());
}
}
private void CollectCoin(GameObject coin) {
Destroy(coin);
score++;
audioSource.PlayOneShot(shique);
}
private void TakeDamage(int amount) {
hp -= amount;
if (hp <= 0) {
Die();
}
}
private void Die() {
Destroy(gameObject);
// TODO: 处理玩家死亡逻辑
}
}
```
阅读全文
相关推荐
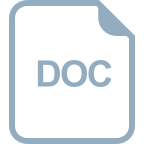
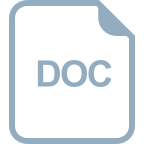
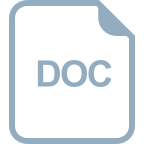


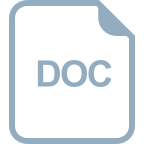
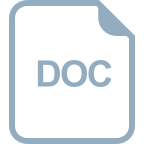
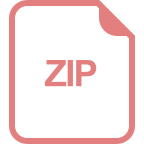
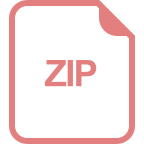
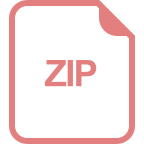
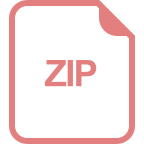
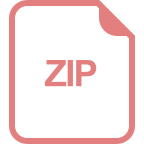