类的继承定义一个computer类在此基础上派生出两个子类(继承与多态绑定)用java语言
时间: 2024-03-02 09:49:45 浏览: 114
好的,下面是一个在Java中实现的示例代码:
```java
class Computer {
private String brand;
private String model;
public Computer(String brand, String model) {
this.brand = brand;
this.model = model;
}
public void displayInfo() {
System.out.println("Brand: " + this.brand);
System.out.println("Model: " + this.model);
}
}
class Laptop extends Computer {
private double weight;
public Laptop(String brand, String model, double weight) {
super(brand, model);
this.weight = weight;
}
@Override
public void displayInfo() {
super.displayInfo();
System.out.println("Weight: " + this.weight);
}
}
class Desktop extends Computer {
private int monitorSize;
public Desktop(String brand, String model, int monitorSize) {
super(brand, model);
this.monitorSize = monitorSize;
}
@Override
public void displayInfo() {
super.displayInfo();
System.out.println("Monitor Size: " + this.monitorSize);
}
}
```
在上面的代码中,我们定义了一个 `Computer` 类,它具有 `brand` 和 `model` 两个私有属性,以及一个 `displayInfo` 方法,用于显示计算机的信息。
然后,我们定义了两个子类 `Laptop` 和 `Desktop`,它们继承自 `Computer`。`Laptop` 类增加了一个 `weight` 属性,并重写了 `displayInfo` 方法,以便能够显示出计算机的重量。`Desktop` 类增加了一个 `monitorSize` 属性,并重写了 `displayInfo` 方法,以便能够显示出计算机的显示器尺寸。
这样,我们就可以创建 `Computer`、`Laptop` 和 `Desktop` 类的对象,并使用它们的方法来显示它们的信息了。具体示例如下:
```java
Computer computer = new Computer("Dell", "OptiPlex 9020");
computer.displayInfo();
Laptop laptop = new Laptop("Lenovo", "ThinkPad X1 Carbon", 1.13);
laptop.displayInfo();
Desktop desktop = new Desktop("HP", "EliteDesk 800 G1", 24);
desktop.displayInfo();
```
输出结果如下:
```
Brand: Dell
Model: OptiPlex 9020
Brand: Lenovo
Model: ThinkPad X1 Carbon
Weight: 1.13
Brand: HP
Model: EliteDesk 800 G1
Monitor Size: 24
```
阅读全文
相关推荐
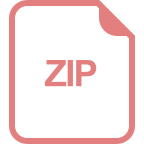
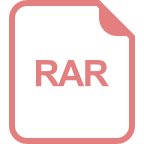
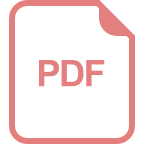
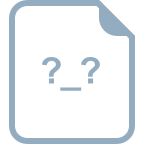
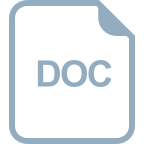
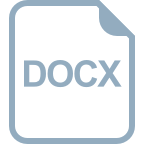
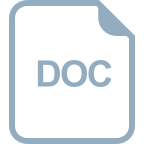
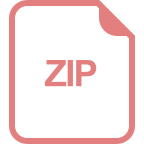
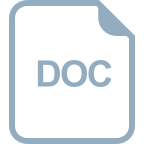
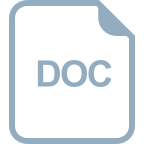

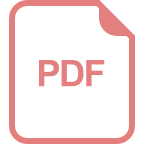
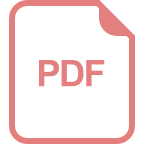
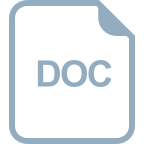