写一段android 把接口返回的文件流保存到本地并于保存完成打开文件所在路径的文件夹的代码
时间: 2024-02-22 09:54:54 浏览: 99
好的,以下是一个实现的示例:
```java
public class SaveFileTask extends AsyncTask<String, Void, Boolean> {
private Context mContext;
private String mFileName;
private InputStream mInputStream;
public SaveFileTask(Context context, String fileName, InputStream inputStream) {
mContext = context;
mFileName = fileName;
mInputStream = inputStream;
}
@Override
protected Boolean doInBackground(String... params) {
boolean result = false;
try {
File dir = mContext.getExternalFilesDir(Environment.DIRECTORY_DOWNLOADS);
if (dir != null && !dir.exists()) {
dir.mkdirs();
}
File file = new File(dir, mFileName);
FileOutputStream fos = new FileOutputStream(file);
byte[] buffer = new byte[1024];
int len;
while ((len = mInputStream.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
fos.flush();
fos.close();
result = true;
} catch (IOException e) {
e.printStackTrace();
} finally {
if (mInputStream != null) {
try {
mInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return result;
}
@Override
protected void onPostExecute(Boolean result) {
if (result) {
Toast.makeText(mContext, "文件已保存到最近文件夹中", Toast.LENGTH_SHORT).show();
openFileDir();
} else {
Toast.makeText(mContext, "保存文件失败", Toast.LENGTH_SHORT).show();
}
}
private void openFileDir() {
Intent intent = new Intent(Intent.ACTION_GET_CONTENT);
Uri uri = Uri.parse(Environment.getExternalStorageDirectory().getPath()
+ "/" + Environment.DIRECTORY_DOWNLOADS);
intent.setDataAndType(uri, "*/*");
mContext.startActivity(Intent.createChooser(intent, "打开文件夹"));
}
}
```
调用方式:
```java
new SaveFileTask(this, "file_name", inputStream).execute();
```
其中,`inputStream`是接口返回的文件流,`file_name`是文件名。以上代码会将文件保存到最近文件夹中,如果该文件夹不存在,则会自动创建。保存成功后会弹出提示并打开文件所在路径的文件夹。
阅读全文
相关推荐
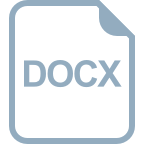
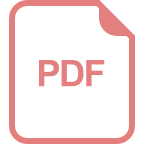


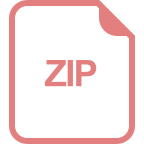
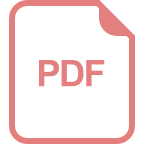
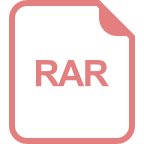
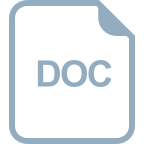
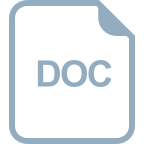








