pyqt5实现简易计算器
时间: 2023-10-30 17:03:17 浏览: 40
以下是一个简单的 PyQt5 计算器实现:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QVBoxLayout, QHBoxLayout, QGridLayout, QPushButton, QLineEdit
import sys
class Calculator(QMainWindow):
def __init__(self):
super().__init__()
# 设置窗口标题和大小
self.setWindowTitle('Calculator')
self.setFixedSize(240, 320)
# 创建中心窗口和布局
self.central_widget = QWidget()
self.setCentralWidget(self.central_widget)
self.central_layout = QVBoxLayout()
self.central_widget.setLayout(self.central_layout)
# 创建文本框和按钮
self.display = QLineEdit()
self.display.setReadOnly(True)
self.central_layout.addWidget(self.display)
self.buttons = []
button_layout = QGridLayout()
button_layout.setSpacing(5)
for i in range(0, 10):
button = QPushButton(str(i))
button.clicked.connect(self.button_clicked)
button_layout.addWidget(button, int((9-i)/3), (i-1)%3)
self.buttons.append(button)
self.add_button = QPushButton('+')
self.add_button.clicked.connect(self.button_clicked)
button_layout.addWidget(self.add_button, 0, 3)
self.sub_button = QPushButton('-')
self.sub_button.clicked.connect(self.button_clicked)
button_layout.addWidget(self.sub_button, 1, 3)
self.mul_button = QPushButton('*')
self.mul_button.clicked.connect(self.button_clicked)
button_layout.addWidget(self.mul_button, 2, 3)
self.div_button = QPushButton('/')
self.div_button.clicked.connect(self.button_clicked)
button_layout.addWidget(self.div_button, 3, 3)
self.dot_button = QPushButton('.')
self.dot_button.clicked.connect(self.button_clicked)
button_layout.addWidget(self.dot_button, 3, 2)
self.eq_button = QPushButton('=')
self.eq_button.clicked.connect(self.button_clicked)
button_layout.addWidget(self.eq_button, 3, 1)
self.clear_button = QPushButton('C')
self.clear_button.clicked.connect(self.clear_display)
button_layout.addWidget(self.clear_button, 3, 0)
self.central_layout.addLayout(button_layout)
def button_clicked(self):
sender = self.sender()
if sender in self.buttons:
self.display.setText(self.display.text() + sender.text())
elif sender == self.dot_button:
if '.' not in self.display.text():
self.display.setText(self.display.text() + '.')
elif sender == self.add_button:
self.display.setText(self.display.text() + '+')
elif sender == self.sub_button:
self.display.setText(self.display.text() + '-')
elif sender == self.mul_button:
self.display.setText(self.display.text() + '*')
elif sender == self.div_button:
self.display.setText(self.display.text() + '/')
elif sender == self.eq_button:
try:
result = eval(self.display.text())
self.display.setText(str(result))
except:
self.display.setText('Error')
def clear_display(self):
self.display.setText('')
if __name__ == '__main__':
app = QApplication(sys.argv)
calculator = Calculator()
calculator.show()
sys.exit(app.exec_())
```
这个计算器有基本的加减乘除、小数点和等于按钮,并且能够处理异常情况。运行这个程序将会出现一个简单的计算器界面。
相关推荐
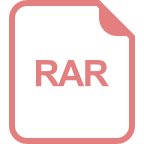
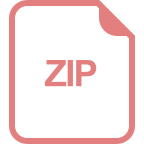
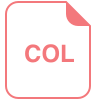
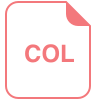
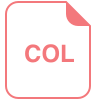
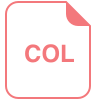
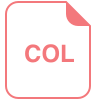









