如何用python将html中的数据存储进mysql中,代码怎么写
时间: 2024-03-13 20:46:55 浏览: 15
首先需要安装 `mysql-connector-python` 库,可以使用以下命令进行安装:
```
pip install mysql-connector-python
```
然后可以使用以下代码将 HTML 数据存储到 MySQL 数据库中:
```python
import mysql.connector
from mysql.connector import errorcode
from bs4 import BeautifulSoup
import requests
# Replace the values below with your actual MySQL database credentials
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'database': 'your_database'
}
# Make a connection to the database
try:
cnx = mysql.connector.connect(**config)
cursor = cnx.cursor()
except mysql.connector.Error as err:
if err.errno == errorcode.ER_ACCESS_DENIED_ERROR:
print("Something is wrong with your username or password")
elif err.errno == errorcode.ER_BAD_DB_ERROR:
print("Database does not exist")
else:
print(err)
# Scrape the HTML data
url = 'https://example.com'
r = requests.get(url)
soup = BeautifulSoup(r.text, 'html.parser')
# Extract the data you need from the HTML
data = soup.find_all('div', class_='my-class')
# Insert the data into the MySQL database
for d in data:
query = "INSERT INTO my_table (column1, column2, column3) VALUES (%s, %s, %s)"
values = (d['data1'], d['data2'], d['data3'])
cursor.execute(query, values)
# Commit the changes to the database and close the connection
cnx.commit()
cursor.close()
cnx.close()
```
在上面的代码中,你需要将 `config` 字典中的值替换为你实际使用的 MySQL 数据库的用户名、密码、主机和数据库名。然后,你需要使用 `requests` 和 `BeautifulSoup` 库来从 HTML 页面中提取数据,将其存储到 `data` 变量中。接着,你可以使用 `INSERT INTO` SQL 语句将数据插入到 MySQL 数据库中。最后,你需要提交更改并关闭数据库连接。
相关推荐
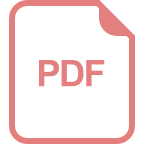
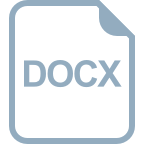
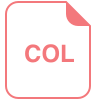
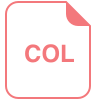
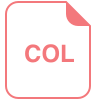
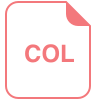
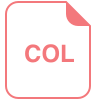









