c语言将txt文本每一行内容赋值给结构体的每行元素
时间: 2023-11-08 07:13:56 浏览: 39
假设结构体定义如下:
```c
#define MAX_LINE_LEN 100
typedef struct {
char content[MAX_LINE_LEN];
} Line;
```
可以按照行读取文本文件,然后将每一行内容分别赋值给结构体的每行元素,代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 100
#define MAX_LINES 100
typedef struct {
char content[MAX_LINE_LEN];
} Line;
int main() {
FILE* fp;
char buf[MAX_LINE_LEN];
Line lines[MAX_LINES];
int i = 0;
fp = fopen("input.txt", "r");
if (fp == NULL) {
printf("Failed to open file\n");
exit(1);
}
while (fgets(buf, MAX_LINE_LEN, fp) != NULL) {
if (i == MAX_LINES) {
printf("Too many lines\n");
exit(1);
}
buf[strcspn(buf, "\n")] = '\0'; // remove trailing newline
strcpy(lines[i].content, buf);
i++;
}
fclose(fp);
// print out the lines
for (int j = 0; j < i; j++) {
printf("Line %d: %s\n", j+1, lines[j].content);
}
return 0;
}
```
其中,`fgets` 函数每次读取一行文本,`strcspn` 函数查找字符串中第一个匹配指定字符集合中的字符,并返回其位置,从而将行末的换行符替换为字符串结束符号。然后,使用 `strcpy` 函数将该行内容拷贝到结构体的 `content` 字段中,最后将结构体数组进行遍历,打印出每一行的内容。
相关推荐
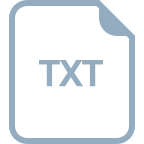
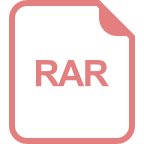
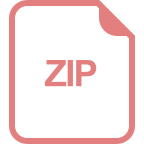














