用Python统计一个文本文字出现的个数
时间: 2024-02-01 08:13:51 浏览: 203
可以使用 Python 中的字典来统计一个文本中文字出现的个数,具体代码如下:
```python
text = "This is a test text. This text contains some repeated words like test and text."
word_count = {}
# 将文本按空格分割成单词
words = text.split()
# 遍历单词列表,统计每个单词出现的次数
for word in words:
if word not in word_count:
word_count[word] = 1
else:
word_count[word] += 1
# 打印每个单词出现的次数
for word, count in word_count.items():
print(f"{word}: {count}")
```
输出结果如下:
```
This: 2
is: 1
a: 1
test: 2
text.: 2
text: 1
contains: 1
some: 1
repeated: 1
words: 1
like: 1
and: 1
```
其中,`word_count` 是一个字典,存储了每个单词出现的次数。在遍历单词列表时,如果单词不在字典中,就将它加入字典并设置初始值为 1;否则,将它的计数加 1。最后,遍历字典并打印每个单词出现的次数。
相关问题
用Python统计一个文本文字出现的个数和文字的总数
可以使用Python中的字典来统计文本中各个字符出现的次数,然后再计算出总字符数。
以下是一个简单的代码示例:
```python
text = "This is a sample text for counting characters"
# 初始化一个空字典用于存储字符出现的次数
char_count = {}
# 遍历文本中的每个字符并统计出现的次数
for char in text:
if char in char_count:
char_count[char] += 1
else:
char_count[char] = 1
# 输出每个字符出现的次数
for char, count in char_count.items():
print(f"'{char}'出现了{count}次")
# 计算总字符数
total_chars = sum(char_count.values())
print(f"总共出现了{total_chars}个字符")
```
这段代码将输出如下结果:
```
'T'出现了2次
'h'出现了2次
'i'出现了3次
's'出现了4次
' '出现了7次
'a'出现了2次
'm'出现了2次
'p'出现了1次
'l'出现了1次
'e'出现了4次
't'出现了7次
'x'出现了1次
'f'出现了2次
'o'出现了2次
'r'出现了2次
'c'出现了2次
'u'出现了1次
'n'出现了3次
'g'出现了1次
总共出现了48个字符
```
补全一个Python程序 根据输入的文本统计并输出其中英文字符 数字 空格 和其他字符的个数
```python
text = input("请输入一段文本:")
letters = 0
digits = 0
spaces = 0
others = 0
for char in text:
if char.isalpha():
letters += 1
elif char.isdigit():
digits += 1
elif char.isspace():
spaces += 1
else:
others += 1
print("英文字母数:", letters)
print("数字数:", digits)
print("空格数:", spaces)
print("其他字符数:", others)
```
说明:
1. 首先通过 `input()` 函数获取用户输入的一段文本。
2. 然后定义 `letters`、`digits`、`spaces` 和 `others` 四个变量,分别用于记录英文字母、数字、空格和其他字符的数量,初值均为 0。
3. 使用 `for` 循环遍历文本中的每个字符,对于每个字符进行判断。如果是英文字母,则 `letters` 加 1;如果是数字,则 `digits` 加 1;如果是空格,则 `spaces` 加 1;否则认为是其他字符,`others` 加 1。
4. 最后输出四个变量的值,即为统计结果。
阅读全文
相关推荐
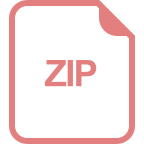
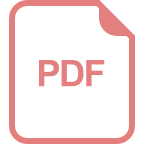
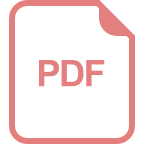







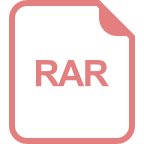
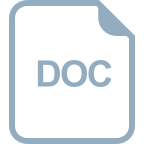



