用python写一个贪吃蛇不用Pygame
时间: 2024-09-29 11:15:00 浏览: 82
当然可以,编写一个简单的Python贪吃蛇游戏并不一定需要Pygame库,因为Python的标准库`turtle`就非常适合用来做这种绘图和动画的练习。下面是一个简化的贪吃蛇游戏示例:
```python
import turtle
import random
# 初始化游戏参数
snake_speed = 0.1
snake_length = 5
snake = [(0, 0)] * snake_length
direction = "right"
food_pos = (random.randint(-280, 280), random.randint(-280, 280))
score = 0
def move_snake():
x, y = snake[-1]
if direction == "up":
y -= snake_speed
elif direction == "down":
y += snake_speed
elif direction == "left":
x -= snake_speed
else:
x += snake_speed
new_head = (x, y)
snake.insert(0, new_head)
# 判断是否吃到食物
if snake[0] == food_pos:
score += 1
grow_snake()
food_pos = place_food(snake_length + snake_speed)
else:
tail = snake.pop()
window.clear()
window.penup()
window.goto(tail)
window.pendown()
def grow_snake():
global snake_length
snake_length += 1
def place_food(length):
while True:
x, y = random.randint(-280, 280), random.randint(-280, 280)
if not any(point == (x, y) for point in snake):
return x, y
def game_loop():
window.listen()
window.onkey(move_up, "Up")
window.onkey(move_down, "Down")
window.onkey(move_left, "Left")
window.onkey(move_right, "Right")
while True:
window.update()
move_snake()
print(f"Score: {score}")
def move_up():
global direction
direction = "up"
def move_down():
global direction
direction = "down"
def move_left():
global direction
direction = "left"
def move_right():
global direction
direction = "right"
# 创建画布并设置初始位置
window = turtle.Screen()
window.bgcolor("black")
window.title("Simple Snake Game")
turtle.speed(0)
turtle.shape("turtle")
turtle.color("white")
turtle.penup()
turtle.hideturtle()
# 开始游戏循环
game_loop()
```
在这个例子中,我们使用了`turtle`模块来绘制屏幕、控制蛇的移动和放置食物。你可以将这个代码复制到一个`.py`文件运行起来,然后通过键盘的方向键控制蛇的行为。
阅读全文
相关推荐
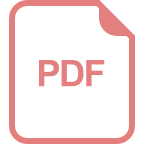
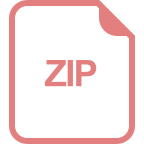




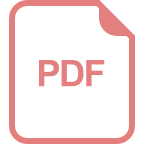