帮我写个linux ptp 使用例程 C语言
时间: 2023-08-29 22:07:28 浏览: 223
好的,下面是一个简单的使用 Linux PTP 实现精确时间同步的例程:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <net/if.h>
#include <linux/ptp_clock.h>
#define PTP_DEVICE "eth0"
int main(int argc, char *argv[]) {
int sock_fd;
struct ifreq ifr;
struct ptp_clock_caps caps;
struct ptp_clock_time time;
struct timespec ts;
// 打开 PTP 设备
sock_fd = socket(AF_INET, SOCK_DGRAM, IPPROTO_UDP);
if (sock_fd < 0) {
perror("socket");
exit(EXIT_FAILURE);
}
memset(&ifr, 0, sizeof(ifr));
strncpy(ifr.ifr_name, PTP_DEVICE, IFNAMSIZ - 1);
if (ioctl(sock_fd, SIOCGIFINDEX, &ifr) < 0) {
perror("ioctl");
close(sock_fd);
exit(EXIT_FAILURE);
}
// 获取时钟能力
if (ioctl(sock_fd, PTP_CLOCK_GETCAPS, &caps) < 0) {
perror("ioctl");
close(sock_fd);
exit(EXIT_FAILURE);
}
// 检查时钟是否支持 Time Stamping
if (!(caps.flags & PTP_CLOCK_CAP_EXTTS)) {
fprintf(stderr, "PTP device does not support Time Stamping\n");
close(sock_fd);
exit(EXIT_FAILURE);
}
// 获取当前时间
if (ioctl(sock_fd, PTP_CLOCK_GETTIME, &time) < 0) {
perror("ioctl");
close(sock_fd);
exit(EXIT_FAILURE);
}
ts.tv_sec = time.sec;
ts.tv_nsec = time.nsec;
printf("Current time: %ld.%09ld\n", ts.tv_sec, ts.tv_nsec);
// 关闭 PTP 设备
close(sock_fd);
return 0;
}
```
该例程首先打开指定的 PTP 设备(这里使用的是 `eth0`),然后获取该时钟的能力,检查是否支持 Time Stamping,并获取当前时间(以秒和纳秒表示)。最后关闭 PTP 设备。需要注意的是,这个例程只是一个简单的示例,实际使用时需要根据需求进行修改和扩展。
阅读全文
相关推荐
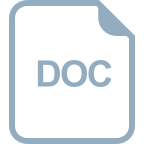
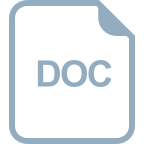
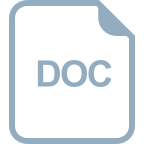
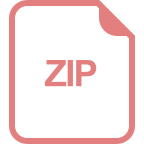
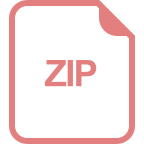
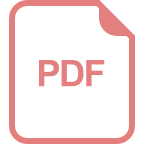
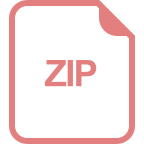
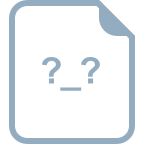
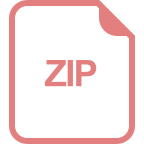








