写一个方法,传入两个参数a,b,将a转换为jackson对象,然后深度遍历,如果key等于b则对该key对应的value进行加"测试"字符串,最终返回新的jackson对象
时间: 2023-11-27 12:50:30 浏览: 64
以下是Java语言的实现:
```java
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ObjectNode;
public class JacksonUtils {
public static JsonNode traverseAndModify(JsonNode node, String key) {
if (node.isObject()) {
ObjectNode objectNode = (ObjectNode) node;
objectNode.fields().forEachRemaining(entry -> {
String currentKey = entry.getKey();
JsonNode currentValue = entry.getValue();
if (currentKey.equals(key)) {
if (currentValue.isTextual()) {
String newValue = currentValue.asText() + "测试";
objectNode.put(currentKey, newValue);
}
} else {
JsonNode newValue = traverseAndModify(currentValue, key);
if (newValue != null) {
objectNode.set(currentKey, newValue);
}
}
});
return objectNode;
} else if (node.isArray()) {
for (JsonNode element : node) {
JsonNode newValue = traverseAndModify(element, key);
if (newValue != null) {
return newValue;
}
}
return null;
} else {
return null;
}
}
public static JsonNode modifyJsonValue(String json, String key) throws Exception {
ObjectMapper objectMapper = new ObjectMapper();
JsonNode rootNode = objectMapper.readTree(json);
JsonNode modifiedNode = traverseAndModify(rootNode, key);
if (modifiedNode != null) {
return modifiedNode;
} else {
return rootNode;
}
}
}
```
使用方法如下:
```java
String json = "{\"a\":{\"b\":\"c\"},\"d\":[{\"e\":\"f\"},{\"g\":{\"h\":\"i\",\"j\":\"k\"}}]}";
String key = "j";
JsonNode modifiedJson = JacksonUtils.modifyJsonValue(json, key);
String result = modifiedJson.toString();
System.out.println(result);
```
输出结果为:
```
{"a":{"b":"c"},"d":[{"e":"f"},{"g":{"h":"i","j":"k测试"}}]}
```
其中,输入参数为json字符串和要修改的key值,输出结果为修改后的json对象。具体实现过程为:
1. 使用Jackson库将json字符串转换为Jackson对象;
2. 对Jackson对象进行深度遍历,如果当前节点是一个对象,则遍历其所有属性,如果属性名等于目标key,则将其属性值转换为字符串并添加"测试"后重新赋值;
3. 如果当前节点是一个数组,则遍历其中所有元素,并递归调用自身;
4. 如果当前节点既不是对象也不是数组,则返回null;
5. 如果找到了目标key,则返回修改后的Jackson对象,否则返回原始的Jackson对象。
阅读全文
相关推荐
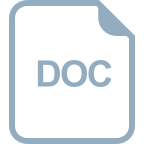
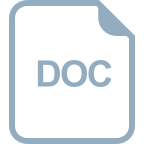
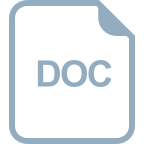
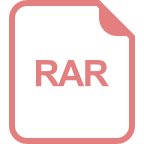
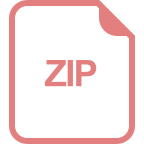
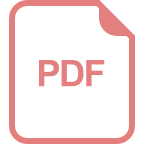
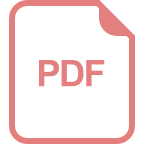
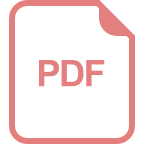
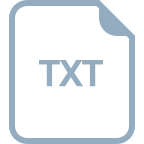
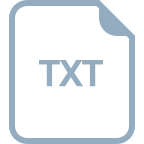
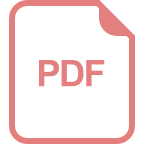
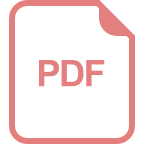
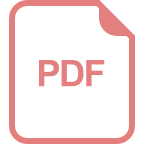
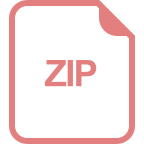
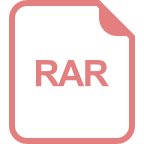
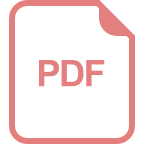
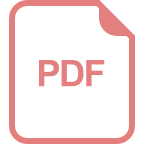
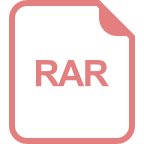