python 类中的的 field:dict[str,dict]有什么作用
时间: 2024-02-13 21:59:05 浏览: 120
在Python类中,field: dict[str, dict]是一个字段(field),它的类型是字典(dict)。这个字段的作用是存储一组键值对,其中键是字符串(str),值是字典(dict)。
通过使用这个字段,我们可以在类中方便地存储和访问多个相关的数据。字典是一种非常灵活的数据结构,可以根据需要动态地添加、删除和修改键值对。在类中使用字典字段可以提供更好的组织和管理数据的方式。
例如,我们可以将field字段用于存储学生信息,其中每个学生的学号作为键,对应的学生信息(如姓名、年龄、成绩等)作为值。通过field字段,我们可以轻松地添加、查找和修改学生信息。
另外,使用字典作为字段还可以实现更复杂的数据结构,例如嵌套字典,可以用于表示树形结构、配置文件等。
相关问题
-- coding: utf-8 -- import arcpy arcpy.env.overwriteOutput = True # 输入参数 input_feature_class = arcpy.GetParameterAsText(0) # 输入要素类 join_feature_class = arcpy.GetParameterAsText(1) # 连接要素类 output_feature_class = arcpy.GetParameterAsText(2) # 输出要素类 join_fields = arcpy.GetParameterAsText(3) # 连接字段 merge_fields = arcpy.GetParameterAsText(4) # 合并字段 min_area = arcpy.GetParameter(5) # 最小面积 # 创建空间连接 arcpy.SpatialJoin_analysis(input_feature_class, join_feature_class, output_feature_class, "JOIN_ONE_TO_MANY", "", "", "INTERSECT") # 创建输出要素类的字段列表 field_list = [f.name for f in arcpy.ListFields(output_feature_class)] # 创建合并字段的字典 merge_dict = {} # 遍历输出要素类中的要素 with arcpy.da.UpdateCursor(output_feature_class, field_list) as cursor: for row in cursor: # 如果要素面积大于最小面积,则进行合并 if row[0] > min_area: # 创建合并字段的键值 merge_key = tuple([row[field_list.index(f)] for f in merge_fields.split(";")]) # 如果合并字段的键值不存在,则添加到字典中 if merge_key not in merge_dict: merge_dict[merge_key] = [] # 将当前要素的连接字段值添加到字典中 join_values = [row[field_list.index(f)] for f in join_fields.split(";")] merge_dict[merge_key].append(join_values) # 删除不符合条件的要素 else: cursor.deleteRow() # 遍历合并字段的字典,将连接字段的值合并并更新到输出要素类中 with arcpy.da.UpdateCursor(output_feature_class, merge_fields.split(";")) as cursor: for row in cursor: merge_key = tuple(row) if merge_key in merge_dict: join_values = merge_dict[merge_key] join_values = ["/".join([str(v) for v in j]) for j in join_values] row = tuple(join_values) cursor.updateRow(row)运行错误:IndentationError: unexpected indent (空间连接.py, line 18) 执行(空间连接多对一)失败。请改正代码
这个错误是缩进错误,你需要检查第18行到23行的代码缩进是否正确。正确的代码应该是这样的:
```python
# 遍历输出要素类中的要素
with arcpy.da.UpdateCursor(output_feature_class, field_list) as cursor:
for row in cursor:
# 如果要素面积大于最小面积,则进行合并
if row[0] > min_area:
# 创建合并字段的键值
merge_key = tuple([row[field_list.index(f)] for f in merge_fields.split(";")])
# 如果合并字段的键值不存在,则添加到字典中
if merge_key not in merge_dict:
merge_dict[merge_key] = []
# 将当前要素的连接字段值添加到字典中
join_values = [row[field_list.index(f)] for f in join_fields.split(";")]
merge_dict[merge_key].append(join_values)
# 删除不符合条件的要素
else:
cursor.deleteRow()
```
另外,你还需要检查代码中是否存在其他的缩进错误。
import arcpy# 输入参数 input_features = arcpy.GetParameterAsText(0) # 连接要素 join_field = arcpy.GetParameterAsText(1) # 连接字段 target_feature = arcpy.GetParameterAsText(2) # 目标要素 target_field = arcpy.GetParameterAsText(3) # 目标字段 area_threshold = arcpy.GetParameterAsText(4) # 面积阈值 # 创建空间连接 arcpy.SpatialJoin_analysis(input_features, target_feature, "in_memory/spatial_join", "JOIN_ONE_TO_ONE", "KEEP_ALL", "", "INTERSECT") # 选择面积大于阈值的连接要素 arcpy.Select_analysis("in_memory/spatial_join", "in_memory/selected_features", "''Shape_Area' > ' + str(area_threshold) # 统计相同连接字段值的面积总和 arcpy.Statistics_analysis("in_memory/selected_features", "in_memory/summarized_features", "Shape_Area SUM", join_field) # 创建字典,存储连接字段值和对应的面积总和 sum_dict = {} with arcpy.da.SearchCursor("in_memory/summarized_features", [join_field, "SUM_Shape_Area"]) as cursor: for row in cursor: sum_dict[row[0]] = row # 更新目标要素中的字段值 with arcpy.da.UpdateCursor(target_feature, [target_field, join_field]) as cursor: for row in cursor: join_value = row[1] if join_value in sum_dict: area_sum = sum_dict[join_value] row[0] = str(area_sum) cursor.updateRow(row) # 导出结果 arcpy.CopyFeatures_management(target_feature, arcpy.GetParameterAsText(5))运行出错:SyntaxError: EOL while scanning string literal (空间连接.py, line 11) 执行(fzzz)失败。请改正代码
根据错误提示,是因为代码中第 11 行字符串结束符缺失。可以尝试在该行末尾添加一个单引号来修复该错误,代码修改如下:
```python
arcpy.Select_analysis("in_memory/spatial_join", "in_memory/selected_features", "''Shape_Area' > '" + str(area_threshold) + "'")
```
另外,代码中还有一个错误,第 22 行的 `row[0] = str(area_sum)` 应该修改为 `row[0] = str(area_sum[1])`,因为 `sum_dict[join_value]` 返回的是一个包含连接字段值和面积总和的元组,用索引 1 取出面积总和才是正确的。修改后的完整代码如下:
```python
import arcpy
# 输入参数
input_features = arcpy.GetParameterAsText(0)
join_field = arcpy.GetParameterAsText(1)
target_feature = arcpy.GetParameterAsText(2)
target_field = arcpy.GetParameterAsText(3)
area_threshold = arcpy.GetParameterAsText(4)
# 创建空间连接
arcpy.SpatialJoin_analysis(input_features, target_feature, "in_memory/spatial_join", "JOIN_ONE_TO_ONE", "KEEP_ALL", "", "INTERSECT")
# 选择面积大于阈值的连接要素
arcpy.Select_analysis("in_memory/spatial_join", "in_memory/selected_features", "''Shape_Area' > '" + str(area_threshold) + "'")
# 统计相同连接字段值的面积总和
arcpy.Statistics_analysis("in_memory/selected_features", "in_memory/summarized_features", "Shape_Area SUM", join_field)
# 创建字典,存储连接字段值和对应的面积总和
sum_dict = {}
with arcpy.da.SearchCursor("in_memory/summarized_features", [join_field, "SUM_Shape_Area"]) as cursor:
for row in cursor:
sum_dict[row[0]] = row
# 更新目标要素中的字段值
with arcpy.da.UpdateCursor(target_feature, [target_field, join_field]) as cursor:
for row in cursor:
join_value = row[1]
if join_value in sum_dict:
area_sum = sum_dict[join_value]
row[0] = str(area_sum[1])
cursor.updateRow(row)
# 导出结果
arcpy.CopyFeatures_management(target_feature, arcpy.GetParameterAsText(5))
```
阅读全文
相关推荐
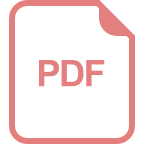
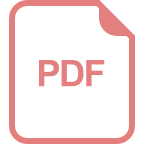
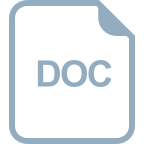
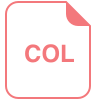
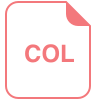
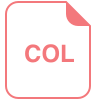
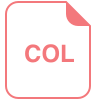
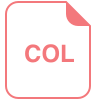
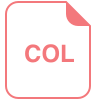
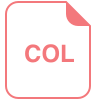
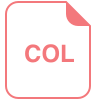
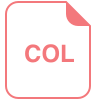
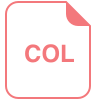
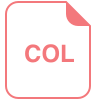
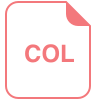

