图像概论实验 编写Python实现直方图均衡化和大津阈值法思路
时间: 2024-10-16 19:18:04 浏览: 67
图像概论实验通常涉及对数字图像的基本处理和分析,其中直方图均衡化是一种常见的增强图像对比度的技术。直方图均衡化主要是为了使图像的像素强度分布更均匀,从而使图像中的细节更加明显。
在Python中,我们可以使用PIL(Python Imaging Library)或其后续版本Pillow库来操作图像,并利用NumPy进行数值计算。以下是直方图均衡化的简单步骤:
1. 导入必要的库:
```python
from PIL import Image
import numpy as np
```
2. 加载图像:
```python
image = Image.open('your_image.jpg')
image_array = np.array(image)
```
3. 计算原图像的直方图(histogram):
```python
hist, bins = np.histogram(image_array.flatten(), 256, [0, 256])
```
4. 创建累积分布函数(Cumulative Distribution Function, CDF),并进行线性插值:
```python
cdf = hist.cumsum()
cdf_normalized = cdf * (255 / cdf.max())
```
5. 使用CDF将每个像素映射到新的亮度值:
```python
equilibrated_array = np.interp(image_array.flatten(), bins[:-1], cdf_normalized).reshape(image_array.shape)
```
6. 再次转换回Image对象并保存结果:
```python
equilibrated_image = Image.fromarray(equilibrated_array.astype('uint8'))
equilibrated_image.save('equilibrated_image.jpg')
```
至于大津阈值法,也称为Otsu's thresholding,它是一种二值化技术,用于自动确定图像中前景和背景之间的最佳分割阈值。Python中可以使用skimage库的`threshold_otsu`函数来应用这种方法:
```python
from skimage.filters import threshold_otsu
# 对图像进行直方图均衡化(如果尚未做)
equilibrated_array = ... # 之前均衡化的数组
# 应用大津阈值
binary_image = equilibrated_array > threshold_otsu(equilibrated_array)
# 保存二值化后的图像
binary_image = Image.fromarray(binary_image.astype('uint8'))
binary_image.save('binary_image.jpg')
```
阅读全文
相关推荐
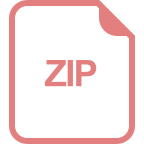
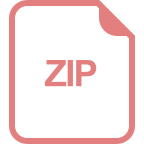















