编写程序,当用户输入某个年份和月份后,判断某年某月有多少天的功能。Python编程
时间: 2024-11-24 11:40:52 浏览: 49
在Python中,我们可以利用内置的calendar模块来计算给定年份和月份的天数。以下是一个简单的示例程序:
```python
import calendar
def get_days_in_month(year, month):
"""
计算并返回指定年份和月份的天数
"""
if 1 <= month <= 12: # 检查月份是否合法
return calendar.monthrange(year, month)[1] # 使用monthrange()函数返回该月的天数
else:
print("无效的月份,请输入1到12之间的整数。")
return None
# 获取用户输入
year = int(input("请输入年份: "))
month = int(input("请输入月份: "))
days = get_days_in_month(year, month)
if days is not None:
print(f"{year}年的{month}月有{days}天。")
```
在这个程序中,`calendar.monthrange()`函数接受年份和月份作为参数,返回一个包含两部分的元组:第一部分是星期几(0表示星期一),第二部分就是该月的天数。如果输入的月份不在1到12之间,程序会提示用户输入无效并返回None。
相关问题
用python编写任务描述 本关任务:编写一个函数,计算某年某月有多少天。 相关知识 31 天的月份有:1、3、5、7、8、10、12 30 天的月份有:4、6、9、11 2 月份:闰年 29 天,平年 28 天 编程要求 根据提示,在右侧编辑器补充代码,输出该月份的天数。 测试说明 平台会对你编写的代码进行测试: 测试输入:2020 1 预期输出: 31 测试输入:2020 2 预期输出: 29
下面是用Python编写的函数,实现计算某年某月有多少天的功能:
```python
def get_days(year, month):
if month in [1, 3, 5, 7, 8, 10, 12]:
return 31
elif month in [4, 6, 9, 11]:
return 30
elif month == 2:
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
return 29
else:
return 28
else:
return -1
```
函数接受两个参数,分别是年份和月份。根据题目给出的要求,判断该月份的天数,并返回结果。如果输入的月份不符合要求,则返回 -1。
可以通过以下测试代码验证函数的正确性:
```python
print(get_days(2020, 1)) # 预期输出:31
print(get_days(2020, 2)) # 预期输出:29
```
编程实现: 从键盘输入年份和月份,计算从该年1月1日到当月月底的总天数。 输入格式: 输入某年某月,如:2021 11 输出格式: 从该年1月1日到当月月底的总天数,最后换行。
在Python中,你可以编写这样的程序来实现这个功能。这里是一个简单的示例:
```python
def calculate_days(year, month):
# 检查输入是否有效
if not (isinstance(year, int) and isinstance(month, int)):
return "无效输入,请输入整数年份和月份。"
# 计算每个月的天数
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0): # 判断闰年
days_in_month[1] = 29
return sum(days_in_month[:month])
# 从用户获取输入
year_str = input("请输入年份(例如:2021): ")
month_str = input("请输入月份(例如:11): ")
year = int(year_str)
month = int(month_str)
result = calculate_days(year, month)
print(f"从{year}年1月1日到{month}月月底的总天数是: {result}\n")
阅读全文
相关推荐




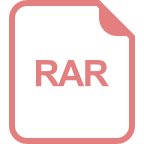









