c++编程实现银行家算法
时间: 2023-12-25 10:29:54 浏览: 69
以下是C++编程实现银行家算法的示例代码:
```cpp
#include <iostream>
#include <vector>
using namespace std;
// 银行家算法函数
bool isSafe(vector<vector<int>>& max, vector<vector<int>>& allocation, vector<int>& available) {
int n = max.size(); // 进程数
int m = max[0].size(); // 资源种类数
vector<vector<int>> need(n, vector<int>(m)); // 需求矩阵
vector<bool> finish(n, false); // 完成标记
// 计算需求矩阵
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
// 模拟分配资源
vector<int> work = available; // 可用资源
vector<int> safeSequence; // 安全序列
int count = 0; // 完成进程数
while (count < n) {
bool found = false;
for (int i = 0; i < n; i++) {
if (!finish[i]) {
bool canAllocate = true;
for (int j = 0; j < m; j++) {
if (need[i][j] > work[j]) {
canAllocate = false;
break;
}
}
if (canAllocate) {
for (int j = 0; j < m; j++) {
work[j] += allocation[i][j];
}
finish[i] = true;
safeSequence.push_back(i);
count++;
found = true;
}
}
}
if (!found) {
break;
}
}
// 判断是否存在安全序列
if (count == n) {
cout << "存在安全序列:" << endl;
for (int i = 0; i < n; i++) {
cout << "P" << safeSequence[i];
if (i != n - 1) {
cout << " -> ";
}
}
cout << endl;
return true;
} else {
cout << "不存在安全序列" << endl;
return false;
}
}
int main() {
// 输入实例数据
vector<vector<int>> max = {{7, 5, 3}, {3, 2, 2}, {9, 0, 2}, {2, 2, 2}, {4, 3, 3}};
vector<vector<int>> allocation = {{0, 1, 0}, {2, 0, 0}, {3, 0, 2}, {2, 1, 1}, {0, 0, 2}};
vector<int> available = {3, 3, 2};
// 调用银行家算法函数
isSafe(max, allocation, available);
return 0;
}
```
阅读全文
相关推荐
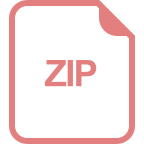
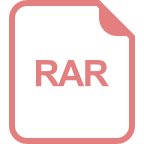
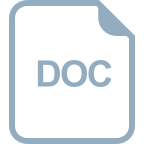
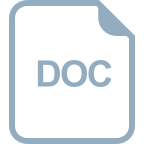
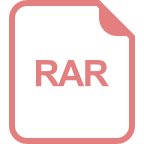
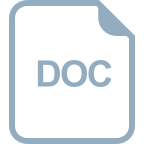
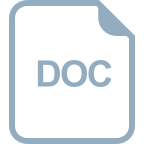
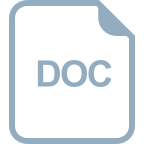
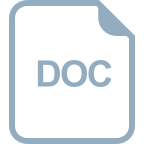
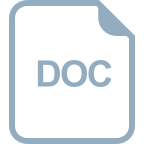
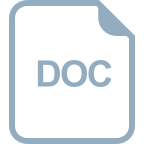
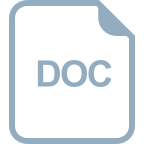
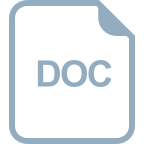
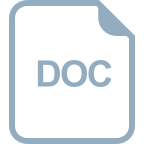
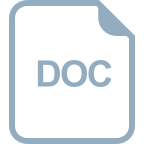
