分析文档并给出相应的代码还有给出思路
时间: 2024-12-22 14:14:45 浏览: 2
### 文档总结与分析
**文档名称**: 实验三:分类实验.docx
**文档类型**: docx
#### 实验概述
- **实验课时**: 4课时,设计性实验
- **实验目的**:
- 理解并掌握逻辑回归分类方法
- 掌握逻辑回归的模型效果评估
- 掌握决策树分类的应用场景
- **实验内容**:
- 对电信客户的流失率进行分析和预测
- 分析用户的套餐、通话、流量、行为、合约、关联购买、使用月数等情况
- 预测用户是否会发生流失
- 后期根据用户情况推出新的政策或活动进行挽留
- **实验流程**:
1. 数据准备
- 数据加载
- 数据分析
- 数据预处理
- 选择特征,划分数据集
2. 预测建模
- 使用决策树、逻辑回归算法在训练集上建模
- 利用测试集得出测试结果
- 使用混淆矩阵和ROC曲线在测试集上评价模型
- **实验成果要求**:
- 完成实验报告,包括实验过程代码和结果分析
- 提交电子档(命名规则:学号+姓名)和纸质档
### 实验代码与思路
#### 1. 数据准备
##### (1) 数据加载
```python
import pandas as pd
# 加载数据
data = pd.read_csv('telecom_customer_data.csv')
print(data.head())
```
##### (2) 数据分析
```python
# 查看数据基本信息
print(data.info())
# 描述统计
print(data.describe())
# 检查缺失值
print(data.isnull().sum())
```
##### (3) 数据预处理
```python
# 处理缺失值
data.fillna(data.mean(), inplace=True)
# 转换类别变量为数值型
data['gender'] = data['gender'].map({'Male': 0, 'Female': 1})
data['Churn'] = data['Churn'].map({'No': 0, 'Yes': 1})
# 剔除无关列
data.drop(['customerID'], axis=1, inplace=True)
```
##### (4) 选择特征,划分数据集
```python
from sklearn.model_selection import train_test_split
# 特征和标签
X = data.drop('Churn', axis=1)
y = data['Churn']
# 划分数据集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
#### 2. 预测建模
##### (1) 使用决策树、逻辑回归算法在训练集上建模
```python
from sklearn.tree import DecisionTreeClassifier
from sklearn.linear_model import LogisticRegression
# 决策树模型
dt_model = DecisionTreeClassifier(random_state=42)
dt_model.fit(X_train, y_train)
# 逻辑回归模型
lr_model = LogisticRegression(max_iter=1000, random_state=42)
lr_model.fit(X_train, y_train)
```
##### (2) 利用测试集得出测试结果
```python
# 预测
dt_predictions = dt_model.predict(X_test)
lr_predictions = lr_model.predict(X_test)
```
##### (3) 使用混淆矩阵和ROC曲线在测试集上评价模型
```python
from sklearn.metrics import confusion_matrix, roc_auc_score, roc_curve, auc
import matplotlib.pyplot as plt
# 混淆矩阵
print("Decision Tree Confusion Matrix:")
print(confusion_matrix(y_test, dt_predictions))
print("Logistic Regression Confusion Matrix:")
print(confusion_matrix(y_test, lr_predictions))
# ROC曲线
def plot_roc_curve(y_true, y_pred, label):
fpr, tpr, _ = roc_curve(y_true, y_pred)
roc_auc = auc(fpr, tpr)
plt.plot(fpr, tpr, label=f'{label} (AUC = {roc_auc:.2f})')
plt.figure()
plot_roc_curve(y_test, dt_model.predict_proba(X_test)[:, 1], 'Decision Tree')
plot_roc_curve(y_test, lr_model.predict_proba(X_test)[:, 1], 'Logistic Regression')
plt.plot([0, 1], [0, 1], color='navy', linestyle='--')
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.05])
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Receiver Operating Characteristic (ROC)')
plt.legend(loc="lower right")
plt.show()
# AUC分数
print("Decision Tree AUC Score:", roc_auc_score(y_test, dt_model.predict_proba(X_test)[:, 1]))
print("Logistic Regression AUC Score:", roc_auc_score(y_test, lr_model.predict_proba(X_test)[:, 1]))
```
### 思路总结
1. **数据准备**:
- 加载数据并查看基本信息
- 处理缺失值和转换类别变量
- 选择特征并划分数据集
2. **预测建模**:
- 使用决策树和逻辑回归算法在训练集上建模
- 在测试集上进行预测
- 使用混淆矩阵和ROC曲线评估模型性能
3. **实验成果**:
- 编写实验报告,包括实验过程代码和结果分析
- 提交电子档和纸质档
希望这些代码和思路能帮助你顺利完成实验!如果有任何问题,请随时提问。
阅读全文
相关推荐
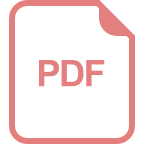
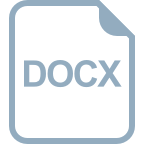
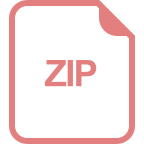
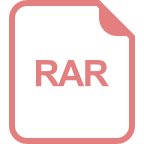
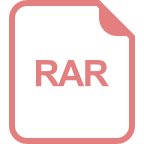
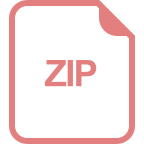
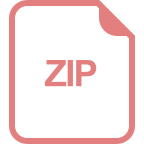
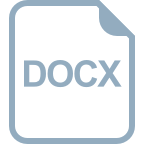
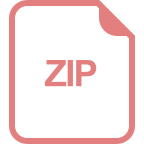
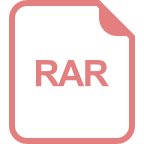
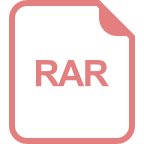
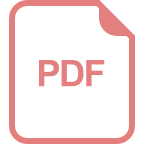
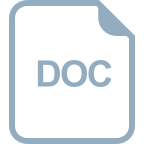
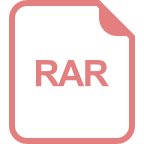
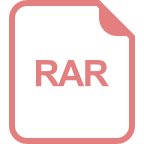
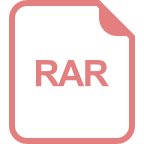
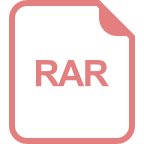
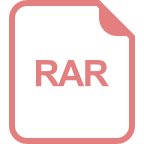
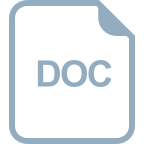