如何使用C++来创建一个顺序表
时间: 2024-09-14 20:14:56 浏览: 41
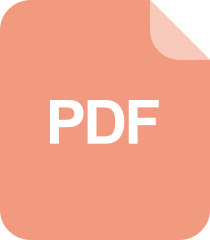
如何在C++中建立一个顺序表

在C++中,创建一个顺序表通常可以通过使用数组或std::vector这两种方式来实现。这里我会提供一个使用数组实现顺序表的示例,以及一个使用std::vector的示例。
首先是使用数组创建顺序表的基本结构:
```cpp
#include <iostream>
template <typename T>
class SeqList {
private:
T* data; // 指向顺序表数据的指针
int capacity; // 顺序表的容量
int length; // 顺序表当前长度
public:
SeqList(int cap = 100) : capacity(cap), length(0) {
data = new T[capacity]; // 动态分配数组
}
~SeqList() {
delete[] data; // 释放数组空间
}
bool insert(int index, const T& value) {
if (index < 0 || index > length || length == capacity) {
return false;
}
for (int i = length; i > index; --i) {
data[i] = data[i - 1]; // 数据后移
}
data[index] = value;
length++;
return true;
}
bool remove(int index) {
if (index < 0 || index >= length) {
return false;
}
for (int i = index; i < length - 1; ++i) {
data[i] = data[i + 1]; // 数据前移
}
length--;
return true;
}
// 其他成员函数,如访问元素、获取长度等,根据需要实现
};
```
使用std::vector创建顺序表的方式则更加简洁:
```cpp
#include <iostream>
#include <vector>
template <typename T>
class SeqList {
private:
std::vector<T> data;
public:
bool insert(int index, const T& value) {
if (index < 0 || index > data.size()) {
return false;
}
data.insert(data.begin() + index, value);
return true;
}
bool remove(int index) {
if (index < 0 || index >= data.size()) {
return false;
}
data.erase(data.begin() + index);
return true;
}
// 使用vector的成员函数,如size(), operator[], at()等访问元素
};
```
阅读全文
相关推荐
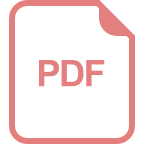
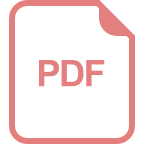
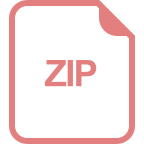
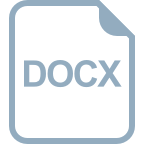
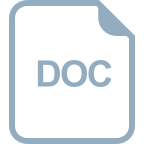
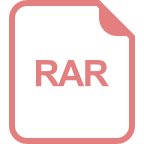
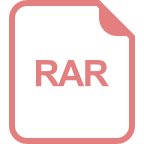
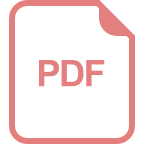
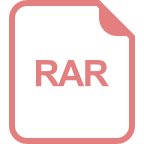
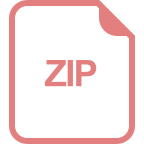
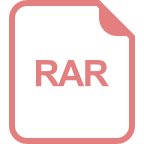
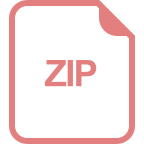
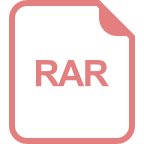
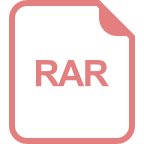
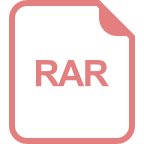
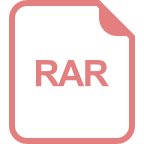
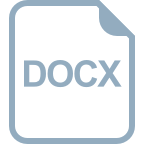