WPF怎么请求API获取天气
时间: 2024-10-16 15:12:38 浏览: 25
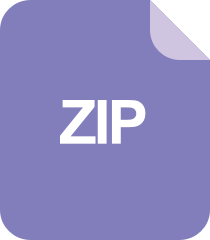
用WPF通过获取天气小软件简单介绍三层架构
在WPF(Windows Presentation Foundation)中,请求API获取天气数据通常涉及到Web服务调用和JSON数据解析。以下是基本步骤:
1. **添加依赖库**:首先,你需要引入一个支持HTTP请求的第三方库,比如`System.Net.Http`(内置)或者NuGet包`RestSharp`或`HttpClientFactory`。
2. **编写请求代码**:
```csharp
using HttpClient httpClient = new HttpClient();
string apiUrl = "https://api.openweathermap.org/data/2.5/weather?q={city name}&appid={your_api_key}";
// 替换 {city name} 和 {your_api_key} 为实际的城市名和API密钥
HttpResponseMessage response = await httpClient.GetAsync(apiUrl);
response.EnsureSuccessStatusCode();
string jsonContent = await response.Content.ReadAsStringAsync();
```
3. **处理响应**:
- 将`jsonContent`作为字符串解析成`WeatherModel`之类的自定义模型,可以使用Json.NET (Newtonsoft.Json) 或 System.Text.Json帮助完成这一步。
```csharp
dynamic data = JsonConvert.DeserializeObject(jsonContent); // 如果使用 Newtonsoft.Json
WeatherModel weatherData = JsonSerializer.Deserialize<WeatherModel>(jsonContent); // 如果使用 System.Text.Json
```
4. **显示天气信息**:
在WPF中,你可以更新UI控件(如TextBlock或Label)来显示天气详情,例如温度、天气状况等。
```csharp
weatherInfoLabel.Content = $"当前温度:{weatherData.main.temp}°C";
```
阅读全文
相关推荐
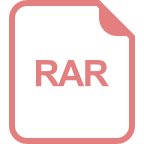
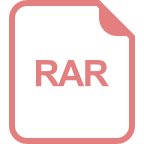
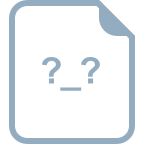
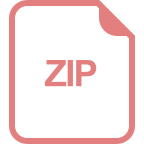
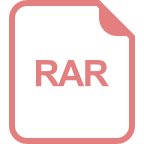
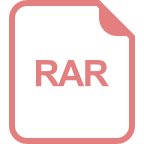
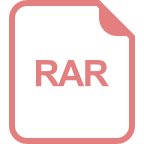
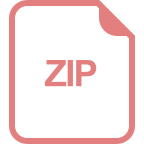
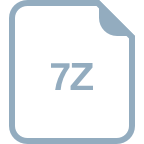
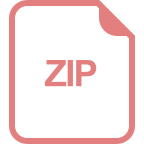
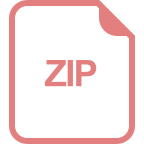
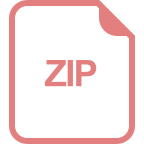
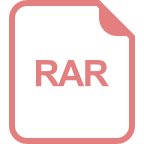
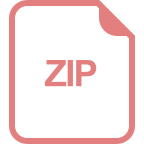
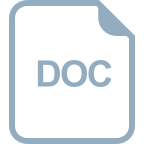
