基于springboot+vue 实现文件上传和下载代码
时间: 2023-09-24 21:06:24 浏览: 101
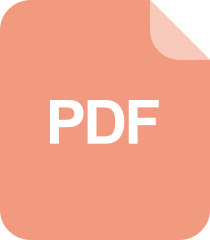
vue+springboot图片上传和显示的示例代码

由于您没有给出具体的需求,以下是一个基本的文件上传和下载的示例代码:
后端代码(基于Spring Boot):
```
@RestController
public class FileController {
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file) {
try {
String filename = file.getOriginalFilename();
byte[] bytes = file.getBytes();
Path path = Paths.get("upload/" + filename);
Files.write(path, bytes);
return "File uploaded successfully!";
} catch (IOException e) {
e.printStackTrace();
return "File upload failed!";
}
}
@GetMapping("/download/{filename:.+}")
public ResponseEntity<Resource> downloadFile(@PathVariable String filename) {
try {
Path path = Paths.get("upload/" + filename);
Resource resource = new UrlResource(path.toUri());
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
} catch (MalformedURLException e) {
e.printStackTrace();
return ResponseEntity.notFound().build();
}
}
}
```
前端代码(基于Vue.js):
```
<template>
<div>
<h2>File Upload and Download</h2>
<input type="file" ref="file" @change="handleFileChange">
<button @click="uploadFile">Upload File</button>
<hr>
<ul>
<li v-for="file in files">
{{file}}
<button @click="downloadFile(file)">Download</button>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
file: null,
files: [],
};
},
methods: {
handleFileChange(event) {
this.file = event.target.files[0];
},
uploadFile() {
let formData = new FormData();
formData.append("file", this.file);
axios.post("/upload", formData).then((response) => {
console.log(response.data);
});
},
downloadFile(filename) {
axios({
url: "/download/" + filename,
method: "GET",
responseType: "blob",
}).then((response) => {
const url = window.URL.createObjectURL(new Blob([response.data]));
const link = document.createElement("a");
link.href = url;
link.setAttribute("download", filename);
document.body.appendChild(link);
link.click();
});
},
},
created() {
axios.get("/list-files").then((response) => {
this.files = response.data;
});
},
};
</script>
```
阅读全文
相关推荐
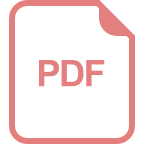
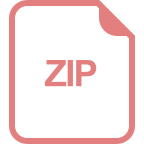
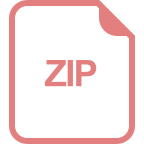
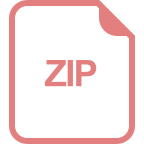
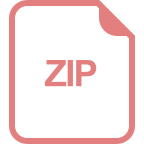
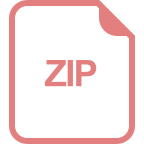
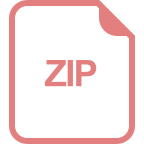
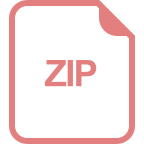
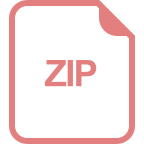
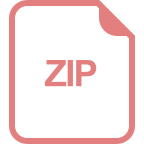
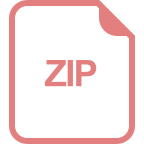
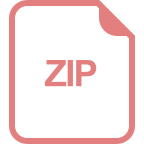
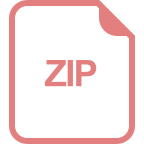
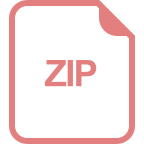
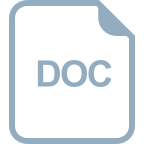
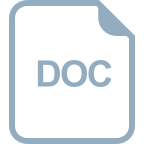
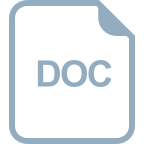