algorithm的内置函数sort
时间: 2023-07-27 13:06:00 浏览: 53
sort()是C++ STL中的一个内置函数,用于对数组或容器中的元素进行排序。它使用的是快速排序算法,时间复杂度为O(NlogN)。
sort()函数的使用格式如下:
```
sort(start, end, cmp)
```
其中,start和end分别表示要排序的元素的起始和结束位置,cmp是可选参数,表示排序时的比较函数,默认按照元素的大小进行排序。
以下是一个示例代码,对一个整型数组进行从小到大排序:
```
#include <iostream>
#include <algorithm>
using namespace std;
int main() {
int arr[] = {5, 2, 3, 1, 4};
int n = sizeof(arr) / sizeof(int);
sort(arr, arr + n);
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
return 0;
}
```
输出结果为:1 2 3 4 5
如果要对一个自定义的结构体进行排序,可以定义一个比较函数来指定排序规则。比较函数的格式如下:
```
bool cmp(const T& a, const T& b)
```
其中,T表示结构体类型,a和b表示要比较的两个元素,函数返回值为bool类型,表示a是否应该排在b之前。
以下是一个示例代码,对一个学生结构体按照分数从高到低排序:
```
#include <iostream>
#include <algorithm>
using namespace std;
struct Student {
string name;
int score;
};
bool cmp(const Student& a, const Student& b) {
return a.score > b.score;
}
int main() {
Student stu[] = {{"Tom", 80}, {"Jerry", 90}, {"Alice", 70}};
int n = sizeof(stu) / sizeof(Student);
sort(stu, stu + n, cmp);
for (int i = 0; i < n; i++) {
cout << stu[i].name << " " << stu[i].score << endl;
}
return 0;
}
```
输出结果为:
```
Jerry 90
Tom 80
Alice 70
```
相关推荐
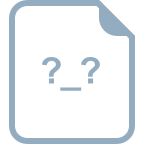
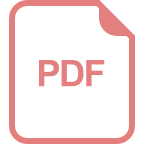
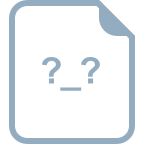














