slam特征点深度 svd_GCNv2-SLAM:用CNN提取特征点取代ORB
时间: 2023-12-06 20:03:58 浏览: 56
感谢您的问题。SLAM中的特征点深度是指相机到地图中的特征点的距离。svd_GCNv2-SLAM是一种使用CNN提取特征点的SLAM方法,它使用图卷积神经网络(GCN)来从图像中提取特征点,然后使用SVD(奇异值分解)来估算它们的深度,以建立地图。与传统基于手工设计的ORB特征点的方法相比,使用CNN提取特征点可以更好地捕捉图像中的结构和纹理信息,从而提高了SLAM性能。
相关问题
orb-slam2特征点提取代码
ORB-SLAM2是一种基于特征点的视觉SLAM系统,它采用ORB特征点作为其特征描述符,下面是ORB-SLAM2特征点提取的核心代码:
```c++
// Extract ORB features from image
void ORBextractor::operator()(cv::Mat image, std::vector<cv::KeyPoint>& keypoints, cv::Mat& descriptors)
{
// Scale the image
cv::Mat image_scaled;
float scale = 1.0f / mvScaleFactors[mvScaleFactors.size()-1];
cv::resize(image, image_scaled, cv::Size(), scale, scale, cv::INTER_LINEAR);
// Compute the ORB features
(*mpORBextractor)(image_scaled, cv::Mat(), keypoints, descriptors);
// Scale keypoint coordinates
if(scale!=1.0f)
{
for(auto& kp : keypoints)
{
kp.pt.x *= scale;
kp.pt.y *= scale;
}
}
}
```
在这个代码中,首先对输入的图像进行缩放,然后调用OpenCV中的ORB特征点提取器对缩放后的图像进行特征点提取,最后再根据缩放因子对提取出的特征点的坐标进行还原。其中,`mvScaleFactors`是一个存储了不同尺度因子的向量,`mpORBextractor`是一个指向ORB提取器的指针。
ORB-SLAM2特征点提取匹配存在的问题
ORB-SLAM2是一种基于特征点的视觉SLAM算法,主要利用ORB特征点进行图像的匹配和姿态估计。虽然该算法在很多场景下表现出色,但是在某些情况下,还是存在一些问题,比如:
1. 特征点分布不均匀。ORB特征点是通过FAST角点检测器和BRIEF描述子生成的,但是在某些场景下,比如低纹理区域或者重复纹理区域,可能无法检测到足够的角点,导致特征点分布不均匀。
2. 特征点匹配错误。由于环境的变化、遮挡等原因,可能会导致特征点匹配错误,从而影响SLAM系统的性能。
3. 运动模糊和图像噪声。在快速运动或者低光照条件下,可能会出现运动模糊和图像噪声,导致特征点提取和匹配的错误率增加。
针对这些问题,可以考虑使用其他的视觉SLAM算法,比如基于直接法的算法,或者结合深度学习的算法,如深度学习视觉SLAM。同时,也可以通过改进ORB-SLAM2的特征点提取和匹配算法,来提高其在复杂场景下的性能。
相关推荐
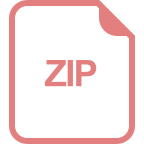
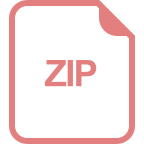












