爬取学校新闻网页的标题,时间,内容,发布人并保存至.csv文件中
时间: 2024-05-04 11:15:24 浏览: 131
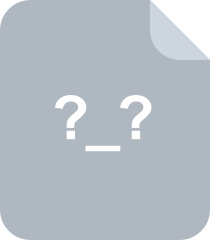
爬取新闻类网页标题和正文
这是一个需要用到爬虫技术的任务,以下是一个基本的思路:
1. 确定目标网站的 URL,比如 http://news.example.com。
2. 使用 Python 的 requests 库向该 URL 发送 HTTP 请求,获取网页内容。
3. 使用 BeautifulSoup 库解析网页内容,提取标题、时间、内容、发布人等信息,并保存到一个字典或列表中。
4. 将这个字典或列表添加到一个总的列表中,每个字典或列表对应一篇新闻。
5. 将总的列表转换为 CSV 格式,使用 Python 的 csv 库将其保存到本地文件中。
以下是一个示例代码,其中需要替换相关变量和函数:
```
import requests
from bs4 import BeautifulSoup
import csv
# 目标网站 URL
url = 'http://news.example.com'
# 发送 HTTP 请求,获取网页内容
response = requests.get(url)
# 解析网页内容
soup = BeautifulSoup(response.content, 'html.parser')
# 提取信息并保存到字典或列表中
news_list = []
for news_item in soup.find_all('div', {'class': 'news-item'}):
title = news_item.find('h2').text.strip()
time = news_item.find('span', {'class': 'time'}).text.strip()
content = news_item.find('div', {'class': 'content'}).text.strip()
author = news_item.find('span', {'class': 'author'}).text.strip()
news_dict = {'title': title, 'time': time, 'content': content, 'author': author}
news_list.append(news_dict)
# 将字典或列表转换为 CSV 格式并保存到本地文件
with open('news.csv', 'w', newline='', encoding='utf-8') as csvfile:
fieldnames = ['title', 'time', 'content', 'author']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for news_dict in news_list:
writer.writerow(news_dict)
```
注意事项:
1. 爬取网站信息需要遵循相关法律法规和网站规定,不得有过多频繁访问网站的行为,否则可能会被封禁或追究法律责任。
2. 代码可能需要根据实际情况进行修改,比如使用不同的 CSS 选择器或正则表达式提取信息,或者添加异常处理等功能。
阅读全文
相关推荐
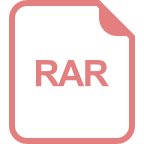
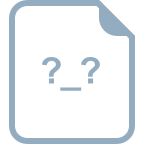
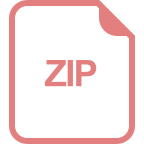
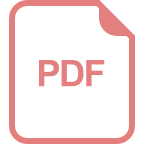
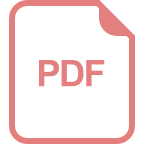
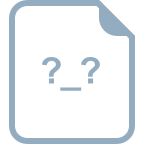
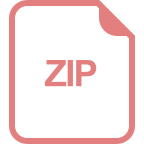
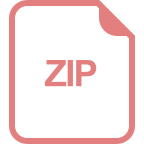
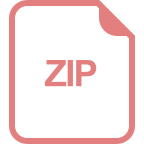
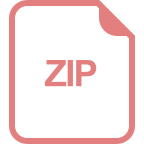
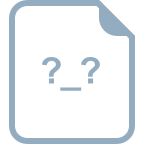
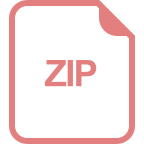
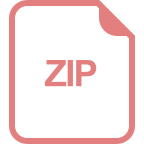
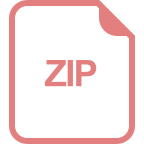
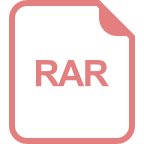
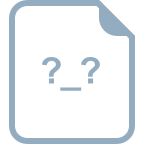