Springboot+mybatisplus如何实现增删改查
时间: 2023-04-12 16:01:12 浏览: 179
C知道:Springboot mybatisplus可以通过使用mybatisplus提供的注解和方法来实现增删改查操作。例如,使用@TableName注解来指定表名,使用@AutoFill注解来自动填充创建时间和更新时间等字段,使用BaseMapper提供的insert、update、delete和select等方法来实现相应的操作。同时,mybatisplus还提供了一些方便的查询方法,如selectList、selectPage等,可以更加方便地进行数据查询和分页操作。至于具体的实现细节,可以参考mybatisplus的官方文档或者相关的教程。
相关问题
springboot整合mybatisplus实现增删改查
### 回答1:
Spring Boot整合Mybatis Plus可以实现快速开发,以下是增删改查的实现方法:
1. 增加数据
使用Mybatis Plus的save方法可以实现数据的添加,例如:
```
User user = new User();
user.setName("张三");
user.setAge(20);
userMapper.insert(user);
```
2. 删除数据
使用Mybatis Plus的deleteById方法可以实现根据ID删除数据,例如:
```
userMapper.deleteById(1L);
```
3. 修改数据
使用Mybatis Plus的updateById方法可以实现根据ID修改数据,例如:
```
User user = new User();
user.setId(1L);
user.setName("李四");
userMapper.updateById(user);
```
4. 查询数据
使用Mybatis Plus的selectList方法可以实现查询所有数据,例如:
```
List<User> userList = userMapper.selectList(null);
```
使用Mybatis Plus的selectById方法可以实现根据ID查询数据,例如:
```
User user = userMapper.selectById(1L);
```
以上就是Spring Boot整合Mybatis Plus实现增删改查的方法。
### 回答2:
SpringBoot是一个快速构建应用的框架,而MybatisPlus是一个简化了Mybatis开发的框架,两者结合可以快速地实现增删改查功能。
1. 准备工作
在pom.xml文件中加入mybatisplus和mysql的依赖:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.2.0</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.22</version>
</dependency>
```
2. 配置文件
在application.properties中配置数据库连接信息和mybatisplus的配置信息:
```properties
spring.datasource.driverClassName=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=UTF-8&serverTimezone=Asia/Shanghai
spring.datasource.username=root
spring.datasource.password=root
# mybatis-plus
mybatis-plus.mapper-locations=classpath*:mapper/*.xml
mybatis-plus.type-aliases-package=com.example.demo.entity
mybatis-plus.configuration.map-underscore-to-camel-case=true
```
3. 实体类
定义一个实体类User,对应数据库中的user表,并使用注解@TableId和@TableName来指定主键和表名:
```java
@Data
@TableName("user")
public class User {
@TableId(type = IdType.AUTO)
private Integer id;
@TableField("username")
private String name;
private Integer age;
}
```
4. Mapper接口
定义一个Mapper接口UserMapper,继承MybatisPlus提供的BaseMapper,无需编写任何SQL语句:
```java
public interface UserMapper extends BaseMapper<User> {
}
```
5. Service层
定义一个Service层的接口UserService,在其中编写增删改查的方法:
```java
public interface UserService {
List<User> list();
boolean save(User user);
boolean updateById(User user);
boolean removeById(Integer id);
User getById(Integer id);
}
```
6. Service实现类
在Service层的实现类UserServiceImpl中注入UserMapper,并实现UserService接口的方法,实现增删改查的功能:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public List<User> list() {
return userMapper.selectList(null);
}
@Override
public boolean save(User user) {
return userMapper.insert(user) > 0;
}
@Override
public boolean updateById(User user) {
return userMapper.updateById(user) > 0;
}
@Override
public boolean removeById(Integer id) {
return userMapper.deleteById(id) > 0;
}
@Override
public User getById(Integer id) {
return userMapper.selectById(id);
}
}
```
7. Controller
在Controller层中注入UserService,并编写接口,调用UserService的方法实现增删改查的功能:
```java
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping
public List<User> list() {
return userService.list();
}
@PostMapping
public boolean save(User user) {
return userService.save(user);
}
@PutMapping
public boolean update(User user) {
return userService.updateById(user);
}
@DeleteMapping("/{id}")
public boolean remove(@PathVariable("id") Integer id) {
return userService.removeById(id);
}
@GetMapping("/{id}")
public User getById(@PathVariable("id") Integer id) {
return userService.getById(id);
}
}
```
至此,SpringBoot整合MybatisPlus实现增删改查的功能已经完成,通过简单的配置和少量的代码,就可以实现数据库操作的功能。
### 回答3:
Spring Boot 是一个开源框架,它使 Spring 应用程序的构建变得更加容易,更加快速,也更加灵活。它提供了一种快速和易于理解的方式来搭建 Spring 应用程序,而 MybatisPlus 是 Mybatis 的一个增强工具,它封装了 Mybatis,提供了很多便捷的功能,如自动生成代码、分页插件等。在实际应用中,用 Spring Boot 整合 MybatisPlus 可以快速地开发高效的数据访问层。
如何整合 Spring Boot 和 MybatisPlus?
首先需要在 pom.xml 文件中添加相关依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- 数据库驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.39</version>
</dependency>
<!-- Mybatis Plus -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>${mybatis-plus.version}</version>
</dependency>
```
在 application.properties 文件中配置数据源和 MybatisPlus:
```properties
# 数据库配置
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8&useSSL=false
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
# 配置 Mybatis Plus
mybatis-plus.mapper-locations=classpath:/mapper/*.xml
mybatis-plus.type-aliases-package=com.example.demo.entity
```
在 Entity 类中使用注解配置,表示表和字段的映射关系:
```java
@Data
public class User {
@TableId(type = IdType.AUTO)
private Long id;
private String name;
private Integer age;
private String email;
}
```
在 Mapper 接口中使用 MybatisPlus 提供的方法,实现数据的增删改查:
```java
@Mapper
public interface UserMapper extends BaseMapper<User> {
}
```
通过继承 BaseMapper 接口,就拥有了 CRUD 的能力,无需写 SQL 语句。在 Service 层中注入 UserMapper,调用相应的方法即可实现对数据的操作:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public List<User> getUserList() {
return userMapper.selectList(null);
}
@Override
public User getUserById(Long id) {
return userMapper.selectById(id);
}
@Override
public int addUser(User user) {
return userMapper.insert(user);
}
@Override
public int updateUser(User user) {
return userMapper.updateById(user);
}
@Override
public int deleteUser(Long id) {
return userMapper.deleteById(id);
}
}
```
在 Controller 中注入 UserService,实现对数据的 RESTful 接口:
```java
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/")
public List<User> userList() {
return userService.getUserList();
}
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getUserById(id);
}
@PostMapping("/")
public int addUser(@RequestBody User user) {
return userService.addUser(user);
}
@PutMapping("/{id}")
public int updateUser(@PathVariable Long id, @RequestBody User user) {
user.setId(id);
return userService.updateUser(user);
}
@DeleteMapping("/{id}")
public int deleteUser(@PathVariable Long id) {
return userService.deleteUser(id);
}
}
```
最后,启动 Spring Boot 应用程序,即可通过浏览器或客户端的 RESTful 接口测试数据的增删改查功能。整个过程较为简单,简化了 Mybatis 的 CRUD 操作步骤,实现了快速开发数据库访问层的目的。
springboot、mybatisplus +vue实现增删改查
好的,针对你的问题,我可以给你讲一下基于SpringBoot和MyBatisPlus开发的Vue增删改查的实现方式。
首先,在SpringBoot中,我们可以通过Spring Data JPA和MyBatisPlus这两个框架来实现数据持久化操作。Spring Data JPA是一种基于注解的继承机制,可以让我们很方便地操作数据库。而MyBatisPlus则是一种基于MyBatis的增强工具,可以在一定程度上简化MyBatis的开发流程。
在具体的实现中,我们可以通过使用MyBatisPlus提供的代码生成器,自动生成数据访问层(Dao)和实体类(Entity),可以省去很多手写代码的繁琐过程。同时,我们也可以根据需要,手动编写Mapper接口和对应的XML文件,进行自定义的操作。
对于前端框架Vue的使用,我们可以先使用VueCLI搭建一个基本的前端工程,然后可以选择使用VueRouter进行路由管理和使用Vuex进行数据状态管理。在具体的页面实现中,我们可以使用Axios等工具进行后端交互,实现增删改查等基本操作。
以上就是基于SpringBoot和MyBatisPlus的Vue增删改查实现方式。希望能够对你有所帮助。
阅读全文
相关推荐
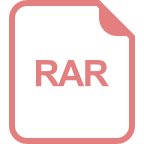
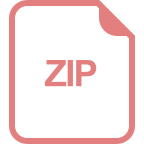
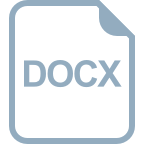



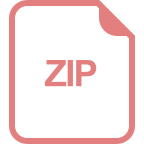
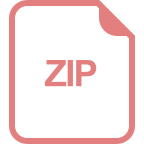
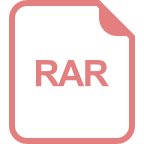
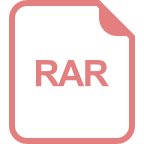
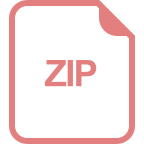
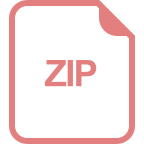
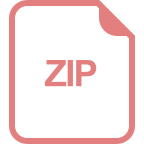

