java实现docx文档模板对表格数据填充并导出
时间: 2024-05-12 09:13:08 浏览: 165
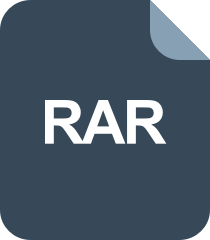
java基于模板导出Excel表格
可以使用Apache POI来实现Java对docx文档的编辑和导出操作,以下是一个简单的示例代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import org.apache.poi.openxml4j.opc.OPCPackage;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.apache.poi.xwpf.usermodel.XWPFTable;
import org.apache.poi.xwpf.usermodel.XWPFTableCell;
import org.apache.poi.xwpf.usermodel.XWPFTableRow;
public class DocxTemplate {
public static void main(String[] args) {
try {
// 读取模板文件
FileInputStream inputStream = new FileInputStream(new File("template.docx"));
XWPFDocument document = new XWPFDocument(OPCPackage.open(inputStream));
// 获取表格并填充数据
XWPFTable table = document.getTableArray(0);
for (int i = 0; i < table.getNumberOfRows(); i++) {
XWPFTableRow row = table.getRow(i);
for (int j = 0; j < row.getTableCells().size(); j++) {
XWPFTableCell cell = row.getCell(j);
String text = "表格数据" + i + "-" + j;
cell.setText(text);
}
}
// 保存文件
FileOutputStream outputStream = new FileOutputStream(new File("output.docx"));
document.write(outputStream);
outputStream.close();
System.out.println("导出成功!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这段代码主要实现了读取一个名为“template.docx”的模板文件,并将第一个表格中的内容替换为指定的数据,最后将结果保存为“output.docx”文件。其中,XWPFTable、XWPFTableRow和XWPFTableCell是POI中表示Word文档中表格、行和单元格的类,通过它们可以方便地访问和修改表格中的数据。
阅读全文
相关推荐

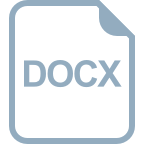
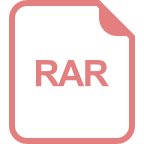
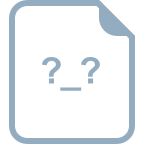
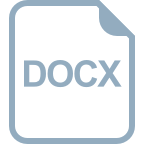
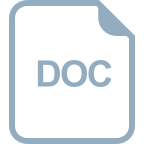


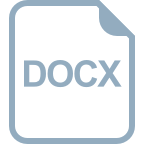
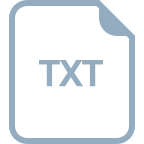
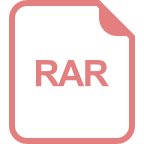
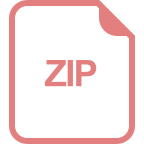
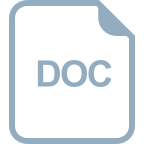
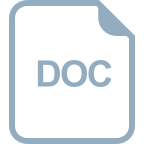
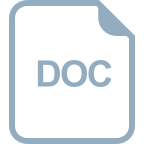