java stream
时间: 2023-09-11 13:12:54 浏览: 90
Java Stream是Java 8中的一种新特性,它允许我们以声明式方式操作集合(List、Set、Map等)和数组等数据源,类似于SQL语句对数据库的操作。Stream可以极大地简化集合的操作,提高代码的可读性和可维护性。
Stream的操作可以分为中间操作和终止操作。中间操作是指对Stream进行转换和过滤等操作,返回一个新的Stream;而终止操作是指对Stream进行聚合、计算等操作,返回一个结果或者副作用。
Stream的优点有:
1. 代码简洁、可读性强,减少了循环和条件判断的代码;
2. 高效,Stream底层采用了惰性求值和短路求值等技术,避免了不必要的计算;
3. 可以并行执行,提高了计算效率。
但是需要注意的是,Stream并不适合所有情况,对于小数据量的集合操作,使用传统的for循环可能更加高效。
相关问题
java Stream
Java Stream是Java 8引入的一个功能强大且易于使用的API,用于对集合(Collection)进行处理和操作。它提供了一种函数式编程风格的方式来处理数据,可以简化代码并提高效率。
Stream可以用于对集合进行过滤、映射、排序、聚合等操作,而无需编写显式的循环。它可以在内部处理数据,并且可以并行执行操作以提高性能。
以下是一些常用的Stream操作:
1. 过滤(Filtering):使用`filter`方法根据指定条件过滤集合中的元素。
```java
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
```
2. 映射(Mapping):使用`map`方法将集合中的元素映射为另一种类型。
```java
List<String> names = Arrays.asList("John", "Mary", "Alice");
List<Integer> nameLengths = names.stream()
.map(String::length)
.collect(Collectors.toList());
```
3. 排序(Sorting):使用`sorted`方法对集合中的元素进行排序。
```java
List<Integer> numbers = Arrays.asList(5, 3, 1, 4, 2);
List<Integer> sortedNumbers = numbers.stream()
.sorted()
.collect(Collectors.toList());
```
4. 聚合(Aggregating):使用`reduce`方法对集合中的元素进行聚合操作。
```java
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.reduce(0, Integer::sum);
```
5. 并行处理(Parallel Processing):使用`parallel`方法将Stream转换为并行流,以并行执行操作。
```java
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.parallelStream()
.reduce(0, Integer::sum);
```
这只是Stream API的一小部分功能,还有更多强大的操作和组合方式可以进行。您可以根据需要使用Stream来处理和操作集合数据,以提高代码简洁性和可读性。
Java Stream
Java Stream is a sequence of elements that supports various operations to perform operations on the data. It is introduced in Java 8 and is used to perform bulk operations on collections of data, such as filtering, mapping, sorting, and reducing.
Streams are designed to work with functional programming concepts, such as lambda expressions and method references. They provide a concise and easy-to-read syntax for performing complex operations on collections of data.
Streams can be created from various sources such as arrays, lists, and collections, and can also be created dynamically using stream builder. Once a stream is created, operations can be performed on it to transform, filter, or aggregate the data.
The two types of operations that can be performed on a stream are intermediate and terminal operations. Intermediate operations return a new stream and allow further operations to be performed on the data. Terminal operations are the final step in the stream pipeline and return a non-stream result, such as a value or a collection.
Java Stream provides several built-in methods for performing common operations on collections, such as filter(), map(), flatMap(), reduce(), and collect(). It also supports parallel processing, allowing operations to be performed on multiple elements concurrently to improve performance.
Overall, Java Stream provides a powerful and flexible way to work with collections of data, allowing developers to write more concise, readable, and efficient code.
阅读全文
相关推荐
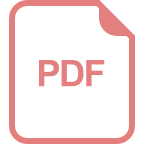
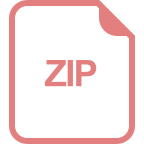
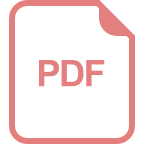










