请帮我用python写一个贪吃蛇
时间: 2024-05-05 18:18:04 浏览: 25
好的,以下是一个基于Python的简单贪吃蛇游戏:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置游戏窗口
width = 500
height = 500
win = pygame.display.set_mode((width, height))
pygame.display.set_caption("贪吃蛇游戏")
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
# 定义贪吃蛇和食物的大小
block_size = 10
# 创建时钟对象
clock = pygame.time.Clock()
# 定义字体
font = pygame.font.SysFont(None, 30)
# 定义贪吃蛇类
class Snake:
def __init__(self):
self.x = width / 2
self.y = height / 2
self.x_change = 0
self.y_change = 0
self.body = []
self.length = 1
def draw(self):
for block in self.body:
pygame.draw.rect(win, black, [block[0], block[1], block_size, block_size])
def move(self):
self.x += self.x_change
self.y += self.y_change
self.body.append([self.x, self.y])
if len(self.body) > self.length:
del self.body[0]
def eat_food(self, food):
if self.x == food.x and self.y == food.y:
self.length += 1
return True
return False
def reset(self):
self.x = width / 2
self.y = height / 2
self.x_change = 0
self.y_change = 0
self.body = []
self.length = 1
# 定义食物类
class Food:
def __init__(self):
self.x = round(random.randrange(0, width - block_size) / 10.0) * 10.0
self.y = round(random.randrange(0, height - block_size) / 10.0) * 10.0
def draw(self):
pygame.draw.rect(win, red, [self.x, self.y, block_size, block_size])
def respawn(self):
self.x = round(random.randrange(0, width - block_size) / 10.0) * 10.0
self.y = round(random.randrange(0, height - block_size) / 10.0) * 10.0
# 定义游戏结束函数
def game_over(score):
message("游戏结束!", white, -50)
message("得分:" + str(score), white, 10)
message("按Q退出,按R重新开始", white, 50)
pygame.display.update()
while True:
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
pygame.quit()
quit()
elif event.key == pygame.K_r:
game_loop()
# 定义信息显示函数
def message(msg, color, y_displace=0):
text = font.render(msg, True, color)
text_rect = text.get_rect()
text_rect.center = (width / 2), (height / 2) + y_displace
win.blit(text, text_rect)
# 定义游戏循环
def game_loop():
snake = Snake()
food = Food()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
snake.x_change = -block_size
snake.y_change = 0
elif event.key == pygame.K_RIGHT:
snake.x_change = block_size
snake.y_change = 0
elif event.key == pygame.K_UP:
snake.y_change = -block_size
snake.x_change = 0
elif event.key == pygame.K_DOWN:
snake.y_change = block_size
snake.x_change = 0
# 移动贪吃蛇
snake.move()
# 判断贪吃蛇是否吃到食物
if snake.eat_food(food):
food.respawn()
# 绘制游戏界面
win.fill(white)
snake.draw()
food.draw()
# 判断贪吃蛇是否碰到了墙
if snake.x >= width or snake.x < 0 or snake.y >= height or snake.y < 0:
game_over(snake.length - 1)
# 判断贪吃蛇是否碰到了自己
for block in snake.body[:-1]:
if block == [snake.x, snake.y]:
game_over(snake.length - 1)
# 刷新界面
pygame.display.update()
# 设置游戏帧率
clock.tick(20)
# 开始游戏
game_loop()
# 退出Pygame
pygame.quit()
quit()
```
希望能够帮助你!
相关推荐



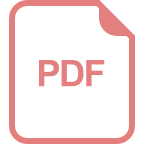
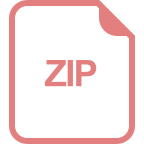