用c语言在屏幕上绘制抛物线与直线的迭加图形。其中抛物线用“*” 表示,直线用“+”表示。抛物线和直线方程自选,但要求该图形带坐标系,且直线斜率可以输入。 用两种颜色区分直线与抛物线。
时间: 2023-06-26 11:09:58 浏览: 125
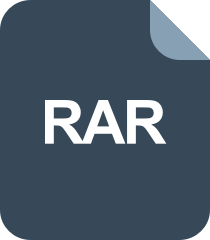
color-parabola-.rar_c语言画抛物线_绘制角度坐标
以下是一份示例代码,用于在屏幕上绘制抛物线和直线的迭加图形:
```
#include <stdio.h>
// 定义屏幕宽度和高度
#define WIDTH 80
#define HEIGHT 40
// 定义坐标系原点
#define ORIGIN_X 40
#define ORIGIN_Y 20
// 绘制坐标系函数
void draw_axes(char canvas[HEIGHT][WIDTH]) {
int i, j;
for(i = 0; i < HEIGHT; i++) {
for(j = 0; j < WIDTH; j++) {
if(i == ORIGIN_Y && j == ORIGIN_X) {
canvas[i][j] = '+';
} else if(i == ORIGIN_Y) {
canvas[i][j] = '-';
} else if(j == ORIGIN_X) {
canvas[i][j] = '|';
} else {
canvas[i][j] = ' ';
}
}
}
}
// 绘制抛物线函数
void draw_parabola(char canvas[HEIGHT][WIDTH]) {
int i, j;
for(i = ORIGIN_Y; i >= 0; i--) {
j = ORIGIN_X - (ORIGIN_Y - i) * (ORIGIN_Y - i) / 4;
if(j >= 0 && j < WIDTH) {
canvas[i][j] = '*';
}
j = ORIGIN_X + (ORIGIN_Y - i) * (ORIGIN_Y - i) / 4;
if(j >= 0 && j < WIDTH) {
canvas[i][j] = '*';
}
}
}
// 绘制直线函数
void draw_line(char canvas[HEIGHT][WIDTH], float slope) {
int i, j;
for(i = 0; i < HEIGHT; i++) {
j = ORIGIN_X + (i - ORIGIN_Y) * slope;
if(j >= 0 && j < WIDTH) {
canvas[i][j] = '+';
}
}
}
// 绘制图形函数
void draw_graph(char canvas[HEIGHT][WIDTH], float slope) {
draw_axes(canvas);
draw_parabola(canvas);
draw_line(canvas, slope);
}
// 显示图形函数
void show_graph(char canvas[HEIGHT][WIDTH]) {
int i, j;
for(i = 0; i < HEIGHT; i++) {
for(j = 0; j < WIDTH; j++) {
printf("%c", canvas[i][j]);
}
printf("\n");
}
}
// 主函数
int main() {
char canvas[HEIGHT][WIDTH];
float slope;
// 输入直线斜率
printf("Enter the slope of the line: ");
scanf("%f", &slope);
// 绘制图形
draw_graph(canvas, slope);
// 显示图形
show_graph(canvas);
return 0;
}
```
在这个示例代码中,我们定义了一个 $80 \times 40$ 的字符画布,用于绘制图形。我们首先通过 `draw_axes` 函数绘制了坐标系,然后通过 `draw_parabola` 函数绘制了抛物线,最后通过 `draw_line` 函数绘制了直线。
在 `main` 函数中,我们首先输入了直线的斜率,然后调用 `draw_graph` 函数绘制图形,最后调用 `show_graph` 函数显示图形。
你可以根据需要修改 `draw_parabola` 和 `draw_line` 函数中的方程,来绘制不同的抛物线和直线。同时,你也可以修改 `draw_graph` 函数中的颜色,来区分直线和抛物线。
阅读全文
相关推荐

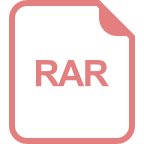
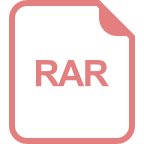
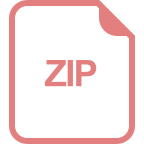
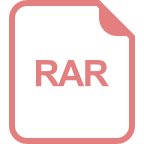
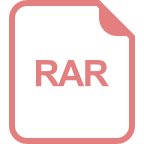
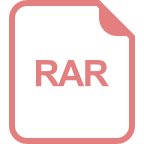
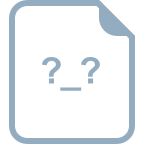
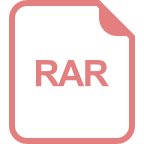
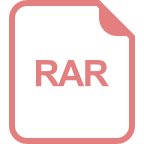
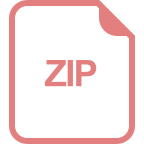
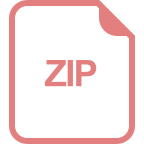
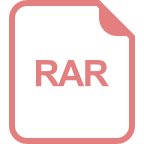


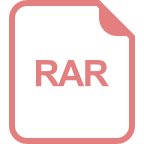