c语言回调函数实现计算器
时间: 2023-09-04 22:09:45 浏览: 53
以下是一个简单的C语言计算器实现,使用回调函数来实现不同的算术操作:
```c
#include <stdio.h>
#include <stdlib.h>
// 回调函数类型,接受两个double类型的参数,返回一个double类型的结果
typedef double (*ArithmeticFunc)(double, double);
double add(double a, double b) {
return a + b;
}
double subtract(double a, double b) {
return a - b;
}
double multiply(double a, double b) {
return a * b;
}
double divide(double a, double b) {
return a / b;
}
int main() {
double num1, num2, result;
char operator;
// 定义一个函数指针变量,用来保存选定的算术操作
ArithmeticFunc arithmeticFunc = NULL;
printf("Enter an arithmetic operation (+, -, *, /): ");
scanf("%c", &operator);
switch (operator) {
case '+':
arithmeticFunc = add;
break;
case '-':
arithmeticFunc = subtract;
break;
case '*':
arithmeticFunc = multiply;
break;
case '/':
arithmeticFunc = divide;
break;
default:
printf("Invalid operator\n");
exit(1);
}
printf("Enter two numbers: ");
scanf("%lf %lf", &num1, &num2);
// 调用回调函数计算结果
result = arithmeticFunc(num1, num2);
printf("Result: %lf\n", result);
return 0;
}
```
该程序使用了函数指针和switch语句来选择要执行的算术操作,并将其保存到函数指针变量中。然后,根据用户输入的两个数字,调用回调函数来计算结果。
相关推荐
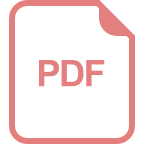
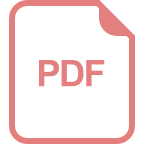
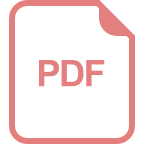














